Since both the REST API and Headless WordPress applications are now mainstream within the WordPress development economy, it’s likely developers have a standard set of tools they like to use when working on these types of projects.
Yours truly not excepted.
And though I’m not making the case that my set of tools should be the standard, I have a set of tools that I’ve found and consistently use when building headless WordPress applications with a REST API.
- MailHog
- Insomnia
- JWT Auth
Though this isn’t in any particular order, I’ll outline them here, how I use them, and explain how they help with login and authentication, testing custom API endpoints, and reviewing emails sent from the local development environments.
Headless WordPress Applications with a REST API
Prerequisites
Before looking at using these tools in an actual project that I’ve been building, it’s worth knowing how I’ve set up my local machine for development. Though you may not do the same, it’s helpful to have some context for what I’m running locally and how your work may diverge or change given your set up.
I’ve documented this in a previous post, but the gist of the tools are as follows:
- Laravel Valet
- MariaDB
- PHP (anywhere between 7.4.33 to 8.2 depending on the server on which a project is running).
You can read about how I’ve set all of the above up locally Installing Valet on macOS with Homebrew.
As far as my development environment is concerned, I also use:
- Visual Studio Code Insiders (which I discuss in this post)
- PHPDoc Comment
- PHP Sniffer & Beautifier with PSR12
- PHP Debug
- Ray
Though all of the above are tools, extensions, or coding styles, I’ve also written extensively about using Ray for WordPress Development.
If you’re familiar with all of the above, have your own set up, or are already comfortable with building applications or solutions in WordPress, then none of the above may be relevant.
Otherwise, take some time to familiarize yourself with everything above and then go from there.
A Brief Case Study
I’ve been working on a headless WordPress application that provides custom endpoints with which an iOS application can communicate to login, authenticate, send, and receive data.
Specifically, the WordPress application provides the following functionality:
- Create a new user account
- Get user information
- Request a token
- Validate a token
- Set data for a user
This is not an exhaustive list but it’s enough for me to demonstrate how the various tools I use can help building similar applications.
Further, endpoints for each of the above look something like this:
/acme/v1/getUser
/acme/v1/createUser
/jwt-auth/v1/token
/jwt-auth/v1/token/validate
/acme/v1/setData
Of course, acme
is a placeholder for the name of my application. jwt-auth
is a namespace for the authenticate library I’m using which is discussed later in this article.
Documenting the API
As with any good API, documentation for an API is key.
First, since we’re developing a headless application, it’s important to think of the REST API and the interface for the application. After all, this is how others are going to interact with the application.
Secondly, looking at the API as a black box helps us to see – as the developer – how others are going to see our application and perhaps uncover any inconsistencies, confusing aspects, or even things we’ve overlooked when building out the API.
In addition to these reasons, there are also the standard reasons that APIs should be documented. This is the case even if an application isn’t seeking some type of mass adoption.
These reasons include:
- clear communication of endpoints, arguments, and expected output,
- ease of which new developers can be onboarded to communicate with the product,
- feedback on how to handle errors in the client application,
- and versioning the API so developers know which version of the API they are using and what features are available when.
There are more but these are points that are consistent across all types of REST API development. And if you’ve never designed, implemented, or added to one before, it’s worth considering each of these when doing so.
MailHog
If you’ve ever interacted with a WordPress installation on a staging
environment or, obviously, a production
environment then you’ve seen the various types of emails that are sent either through interaction with the frontend or with the CLI (via WP CLI).
In development
environments and depending on your set up, it’s a little different when trying to manage these emails (be it just receive them or if you want to customize them through various hooks).
This is where MailHog comes in handy.
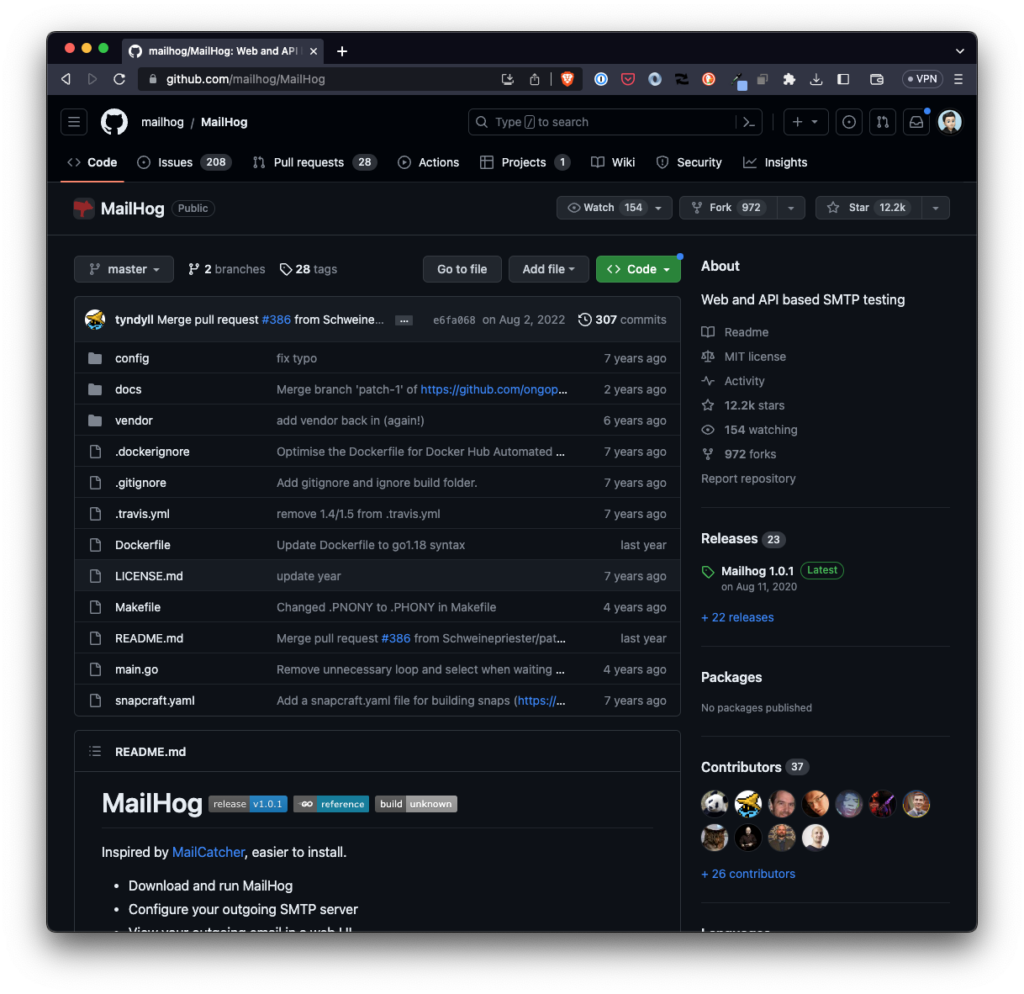
MailHog is a web application is useful when you’re deep in the development and testing stages of your project. It serves as a pseudo-SMTP server, essentially playing the role of a fake email server to help you test and debug email functionality.
Essentially, MailHog intercepts outgoing emails from your application instead of dispatching them to actual recipients. It then captures the email and presents them within a web interface. It then makes it easy to read, parse, and review outbound messages from WordPress.
It allows you to look at the full aspects of outgoing email, too. This includes:
- subject,
- sender,
- recipient,
- and the message.
Ultimately, it makes it much easy to validate email functionality across various environments, and confirming that your emails are correctly formatted and delivered.
And even though this is something that’s useful when working directly within WordPress, it’s also useful to see how the core application is responding in a headless environment especially because everything is being triggered through API endpoints.
Insomnia
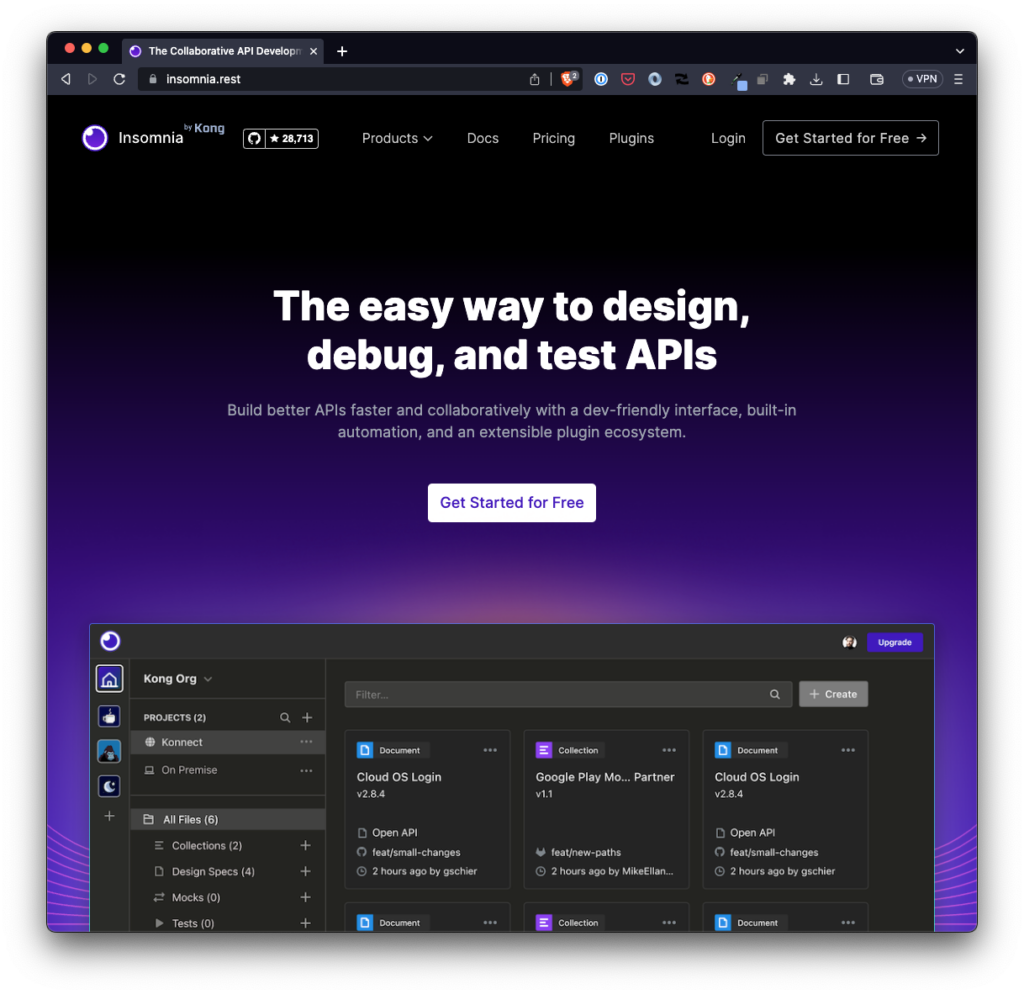
I’ve written about Insomnia before both here and here. If you’re familiar with other REST API clients such as Postman or Paw (now called RapidAPI), then Insomnia should make sense though it’s a bit more lightweight than the aforementioned clients.
For those who aren’t familiar with REST API clients, they all have the same general purpose: to simplify the process of interacting with and testing REST APIs.
Like most REST API clients, Insomnia makes it easy to create, send, and analyze HTTP requests to API endpoints using the standard GET
, POST
, PUT
, and DELETE
actions.
It also makes it easy to work with JSON, XML, and form data. When building your own application, this isn’t as big of a deal because you can control the type of output that will be returned but if you’re interacting with another API (or having one API interact with WordPress) you may need to manipulate data in one format before sending it or receiving it in another format.
One of the notable features of Insomnia is its ability to organize requests into workspaces and folders, allowing developers to keep their API testing projects well-structured and manageable. This makes it simple to navigate and switch between different endpoints and collections.
Additionally, the way the app allows us to document and organize each endpoint not only makes it easy to know what each request should do, but it makes it easy to share with others so they can test it on their own when implementing it in their client.
For example, once I’ve built the REST API for an application that interacts with WordPress, I can share the environment in Insomnia with an iOS developer so they can see:
- how an endpoint is structured,
- what arguments it expects,
- what it returns,
- and the documentation for each endpoint just in case something shows up that’s unexpected.
Ultimately, it’s this type of functionality helps streamline the development process when working with client application developers.
JWT Auth
Before looking into why the JWT Auth plugin is useful, it’s important to understand what JWT – or Java Web Tokens – are and how they are useful in REST API development.
Generally speaking, you’re likely to read see JWT defined as something like:
A way to securely transmit information between parties as JSON objects. In simple terms, JWT is a token-based authentication mechanism commonly used in web applications.
And while this is true, if you’re unfamiliar with exactly how this impacts REST API authentication then it still doesn’t help build a case as to why you need it.
When trying to draw an analogy between how we interact with WordPress in the browser and how we interact with WordPress through JWT look at it this way:
- In the browser, we pass our credentials to the browser and then the application sends data back to the browser. Then we’re able to “walk around” inside of WordPress and perform whatever action our capabilities allow us to do (sometimes to manage users, edit posts, manage taxonomies, and so on; other times, it’s review posts and that’s it). But all of this is managed within the WordPress administration area.
- When you authenticate with WordPress via REST APIs, there needs to be another way to authenticate your action so that the application knows each time you’re hitting an endpoint – or making a request – that you are who you say you are. And that’s where the token comes into play. When you authenticate with WordPress via the REST API, the JWT authorization mechanism will issue you a token. Then, you include that token with each subsequent request. If it expires, the API will let you know and you can refresh your token.
With that said, there’s obviously a bit of heavy lifting that comes with implementing JWT in WordPress. But the JWT Auth plugin makes this much easier.
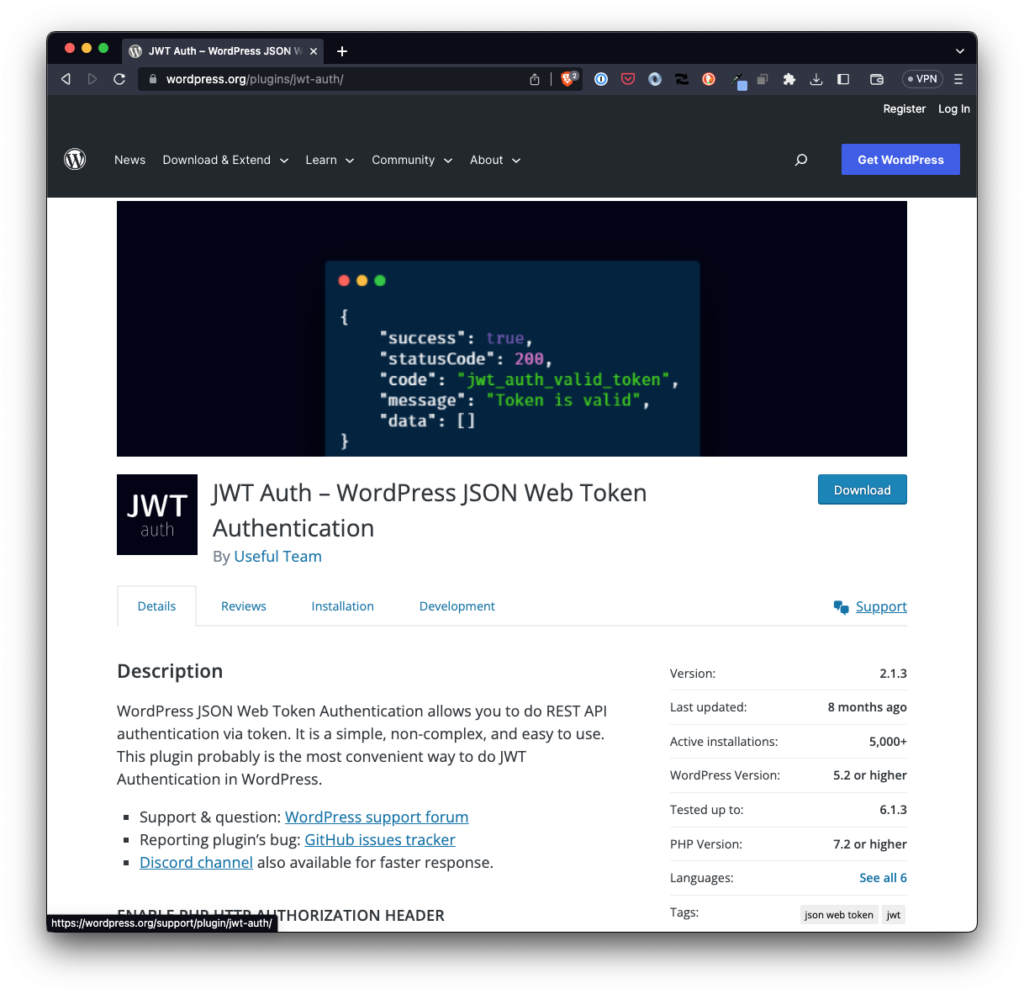
From the plugin page:
WordPress JSON Web Token Authentication allows you to do REST API authentication via token. It is a simple, non-complex, and easy to use. This plugin probably is the most convenient way to do JWT Authentication in WordPress.
JWT Auth – WordPress JSON Web Token Authentication
After trying a number of different plugins this is the one I consistently use. To get this set up, all you need to do is:
- Set a secret key in
wp-config.php
- Determine whether or not you want to support CORS (and this matters if you want to enable secure cross-origin communication between web applications).
After that’s set up, you can simply make a request to /wp-json/jwt-auth/v1/token
given the account’s username and password in a JSON format in the body, like this:
{
"username": "John@appleseed.com",
"password": "kn6oLrcW0\/D1M"
}
And you’ll receive a response that looks like this:
{
"success": true,
"statusCode": 200,
"code": "jwt_auth_valid_credential",
"message": "Credential is valid",
"data": {
"token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpc3MiOiJodHRwczovL3NjYXJpZi50ZXN0IiwiaWF0IjoxNjg0MzU1MzA5LCJuYmYiOjE2ODQzNTUzMDksImV4cCI6MTY4NDk2MDEwOSwiZGF0YSI6eyJ1c2VyIjp7ImlkIjoyNTksImRldmljZSI6IiIsInBhc3MiOiJiMWM3OTBhMmJhYzExNzNlYzI4ZTQxZGUxMDYyYzIwZiJ9fX0.q3-DRC_DQvezy1w-XV0CYNQYyKC3X8iSs7GKy86zjbg",
"id": 259,
"email": "John@appleseed.com",
"nicename": "johnappleseed-com",
"firstName": "John",
"lastName": "Appleseed",
"displayName": "John@appleseed.com"
}
Then you include the token
with each subsequent request and WordPress and the JWT Auth plugin will either allow you to make the request or will block you from doing so.
That’s the gist of how JWT works within headless applications, though. An entire series of articles can be written on how to implement and use this, though. But that’s not this article 🙂.
Conclusion
Building headless WordPress applications with REST APIs offers a powerful approach to creating decoupled services. Tools like:
- Laravel Valet,
- MariaDB,
- and PHP
Provides a solid development environment (if you don’t have one already).
Additionally, tools such as MailHog, Insomnia, and JWT Auth enhance the development process by facilitating email testing, API debugging, and secure authentication.
By adopting a headless architecture, we, as developers, can leverage the flexibility of WordPress as a content management system while utilizing modern technologies for anything ranging from front-end development to iOS development. Ultimately, this separation grants the ability to create custom implementations across various platforms.
Regardless of what you use for your development environment, note:
- MailHog serves as a valuable tool for testing and debugging email functionality
- Insomnia aids in efficient API testing and response analysis,
- JWT Auth enables secure and reliable authentication.
These are the tools I’ve found useful when building headless WordPress applications with a REST API.