Up to this point, everything we’ve talked about regarding the WordPress Settings API and saving data to the database has been based around two ideas:
- We need to sanitize the input
- We haven’t been concerned with any particular fields being required
At this point though, we’re ready to start talking about how to tackle this particular issue. Since we’re already familiar with how to sanitize the data, we’re going to build off of the code that we already have.
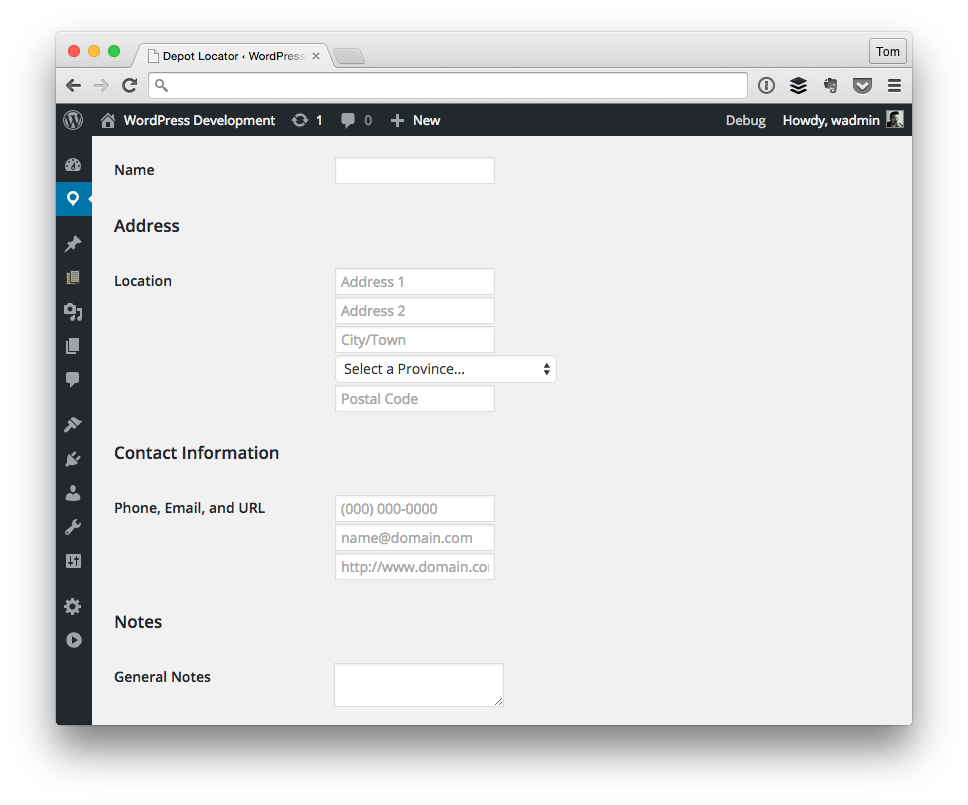
An Example Settings Page
That is, we’re going to look at how to validate the following fields:
- Address 1
- City
- Postal Code
Furthermore, we’re going to see how to tackle this from an object-oriented approach, we’re going to look at what’s needed in order to add an error message if the required input is invalid, and we’re also going to look at how to prevent saving information to the database if it’s empty or invalid.
Object-Orientation and The WordPress Settings API
When it comes to writing code for WordPress, there are some people who lean heavily in the direction of object-oriented programming, there are those who lean heavily into procedural programming, and then there are those who tend to fall in the middle.
Personally, I tend to lean in the direction of object-oriented programming, though this doesn’t mean I avoid procedural programming all together (it’s hard to do that in WordPress, anyway). But for the purposes of this article, I’m going to be tackling the solution using object-oriented programming.
With that said, in order to begin validating the various input fields, it’s important that we identify the main tasks a validation class would do:
- Examine the input
- Determine if it’s valid or not
- If it’s not, then add an error message and prevent it from saving
- If it is, then carry on (or, basically, don’t do anything)
And since we’re validating different types of data (that is, addresses, postal codes, etc.), this means that we’re likely going to have a number of different classes.
Since, however, each class is responsible for the aforementioned tasks, it means that we can define an interface that each class can implement. Let’s do that first:
Remember that an interface can only include public
methods (so adding private
methods to your interface will throw a fatal error and prevent the interpreter from continuing to execute your code). This doesn’t mean that we can’t break our code down into the four steps, though.
Anyway, simple, isn’t it (if not, leave a comment)? So let’s actually implement this interface for the classes responsible for validating each of the above fields.
Addresses
For purposes of validating an address, we only care if the input that the user provides is not empty. As such, the validation logic is really simple.
The key things to take away from this particular class is that is evaluates the input, checks to see if it matches the rule set that we’ve defined, and if it doesn’t then it adds an error message and marks the input as invalid.
This boolean will come back into play in a future article when we look at how we save information (or avoid saving information) to the database.
Cities
Evaluating a city can be as simple or as complicated as you like. In the code below, you’re going to notice very similar functionality as to what is done against addresses above.
The thing is, this is a basic implementation. For example, you may actually want to have a dictionary of sorts – perhaps some type of array – of cities that are valid and then you want to check to see if the specified city is in the array (which can easily be done with in_array
).
Or maybe you just want to check the spelling or formatting of the city. Whatever the case, here’s arguably the most basic case that you can provide:
It’s basically the same as the address code, isn’t it? Again, this is just the base case for how to validate a city. There’s a number of other ways to do it many of which can – and likely are – far more complicated than what I’ve shared here.
Postal Codes
When it comes to validation, postal codes can be a little bit different. For example, perhaps you want to make sure it fits within a set list of valid postal codes (which can again be done with something like in_array
or querying against a database that contains a list of valid codes) or you want to validate against a certain format.
In our case, we’ll be checking that the specified input matches the format of Canadian postal codes. We’ll be using preg_match
to do this. In short, if the format of the input matches that of a regular express, then the postal code will be considered valid.
If, on the other hand, the input isn’t of a valid format, then we’ll mark the input as false and generate an error message.
A Word About Validation
Note that the above code is not necessarily the way to go about validating your input. There are multiple ways that you could go about doing this.
For example, the way that you define your rules for something such as, say, postal codes may vary by country. Further more, you may opt to abstract the error message code into its own class in order to further decrease redundancy.
Where you opt to draw the lines on things like that are a matter of the architecture of what you’re building and your own personal opinion. Each approach has its own pros and cons, but the above is a sufficient starting point especially if this is your first foray into object-oriented programming (let alone validation).
Putting It All Together
In the next post in the series, we’ll look at how to tie everything together so that the information being provided by the user will be sanitized, validated, and then handled appropriately based on everything covered here.
Series Posts
- Sanitizing Multiple Values with the WordPress Settings API
- Sanitizing Arrays: The WordPress Settings API
- On Pause: The WordPress Settings API
- Refactoring Input Sanitization with The WordPress Settings API
- Validating Input via the WordPress Settings API
- Validation and Sanitization in the WordPress Settings API
Leave a Reply
You must be logged in to post a comment.