I’ve talked about using WordPress as a tool for rapid application development in a past article.
But the longer I work with WordPress and the more code I see, the more potential I realize it has as both a platform for rapid prototyping and then taking those prototypes to fully developed applications.
These plugins can be web applications, plugins, themes, websites, whatever. For the purposes of this post, it doesn’t matter. Instead, what matters is that for example:
- you have an idea for a plugin,
- you want to see how it might work within WordPress,
- you quickly put something together,
- you begin refining it.
For many who are getting involved for more serious WordPress development, I thought it might be worth taking a look at what this looks like. Namely, I’ll take an idea for a plugin, prototype it, and then refine it into a well-organized, object-oriented plugin.
So in the next series of articles, I’m going to walk through that process.
Rapid Prototyping with WordPress: The Concept
Though this isn’t always the case, the initial concepts for, say, a plugin will come out of a need for something that you may want for yourself or for someone else.
If you’re like me, it helps to sketch out some initial ideas as to how it might work and make any notes necessary that will help in development.
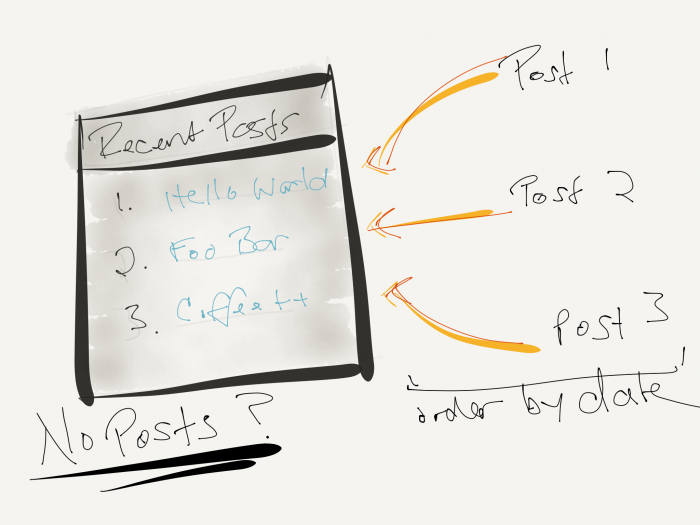
My sharp design skills for the prototype.
Of course, for others, they may opt to jump right into the IDE and begin working on the code. And since, at this phase, it’s just a rapid prototype, there’s nothing wrong with that.
As you can see from the sketch above, I’m looking to write a small plugin that will display my three most recent posts in a meta box.
The idea behind the plugin is this:
- When I’m writing a post, having easy reference to my three most recent posts will give me the ability to easily link to them when wanting to social share my content.
Maybe the purpose of the plugin seems silly; maybe not. Remember that whatever it is you choose to build should be something that’s beneficial both to you and your audience. If someone else doesn’t get it, that’s fine.
Starting The Process
Now that we have a basic idea in place, we know that we’re going to need a few things:
- The plugin file for displaying in the WordPress Plugin screen,
- A meta box for displaying the posts,
- A query for retrieving the posts,
- A way to display a message if there are no posts to display.
Through the the use of a few basic hooks and WordPress APIs, we can put together something, ahem, rapidly. Here’s how:
The Plugin Header
Remember that the plugin header is responsible for displaying the content of the plugin – it’s title, description, version, and author – on the plugin screen. Make sure it’s concise, descriptive, and to the point.
Once this is written, the plugin will appear within the WordPress administration area, but it won’t actually do anything. So let’s give the plugin some actual functionality.
The Meta Box
First, we need to register a meta box. We can use the add_meta_boxes hook for this. It’s easy to define and wire up on our meta box. Take note of the comments for each of the method arguments.
Notice almost that we have a function responsible for displaying information. We have to define this otherwise WordPress will attempt to fire a function that doesn’t exist and this will result in an error.
Let’s define that function now:
Now if you notice, this function relies on three different functions to help it do its work. So we also need to define those functions. Ideally, each of these functions should have a function purpose, however given that we’re rapidly prototyping this project, we may have a bit more work per function than usual.
Querying For Posts
First, we need to query for posts. To do this, we’ll take advantage of WP_Query.
Notice that we return the instance of the query object. We’ll do more with this momentarily.
Displaying a Result
If there are posts to display, then we’ll use a function to iterate through the posts the instance of WP_Query found and render the permalink and title in an ordered list.
Note that this will list up to three, but not necessarily a total of three.
Displaying No Result
If there are no results, then we need to display a message to the user that the query found no posts. The code may look something like this:
Of course, you can customize the message for this all on your own.
Rapid Prototyping, Done.
At this point, there’s a fully-functionality plugin. It’s not without it’s problems, though:
- We’re mixing HTML with our PHP which results in tighter coupling than necessary,
- The HTML is not sanitized or secure in anyway,
- There’s absolutely no object-orientation for these functions which, although some may argue is unnecessary, makes this highly un-modular.
But the point of this series is to take a rapid prototype and move it into a more professional-grade plugin. So we’ve done the first part and now it’s time to start moving into the second phase.
In the meantime, you can follow along with the development of this plugin on GitHub. I’ll be tagging each release that corresponds to a blog post. So this particular post can be found in the 0.1.0 tag.
The master branch will also include the latest version of all merged code and the develop branch will include the code I’m working on but is not complete (nor stable).
And I’ll start doing that in the next post.
Leave a Reply
You must be logged in to post a comment.