The majority of the time that I’m working on a contract project, there’s a need to programmatically create several parts of the website that occur during theme setup. Often times, this includes creating posts, users, and/or setting templates.
Ultimately, the goal is to make the user’s experience as nice as possible: They install the theme and the site, to a degree, has bootstrapped itself.
As with the aforementioned parts of a site, it’s also common to need to create a category, or categories, during theme setup. WordPress provides two ways of doing so, one of which often results in a PHP fatal error.
Here’s how to programmatically create categories in WordPress and do so without generating any errors.
On Inserting Categories
A quick Google search will likely lead you to the Codex article for wp_insert_category
. At first glance, this looks to be exactly what’s needed to programmatically create categories.
Using this particular API function looks something like this:
function example_insert_category() { wp_insert_category( array( 'cat_name' => 'Example Category', 'category_description' => 'This is an example category created with wp_insert_category.', 'category_nicename' => 'example-category', 'taxonomy' => 'category' ) ); } add_action( 'after_setup_theme', 'example_insert_category' );
Clear enough, right? The function accepts an array of arguments that are used to create the category.
If you define this function in your theme (or somewhere in a plugin), then load it up in a browser, you’re likely to see the following screen:
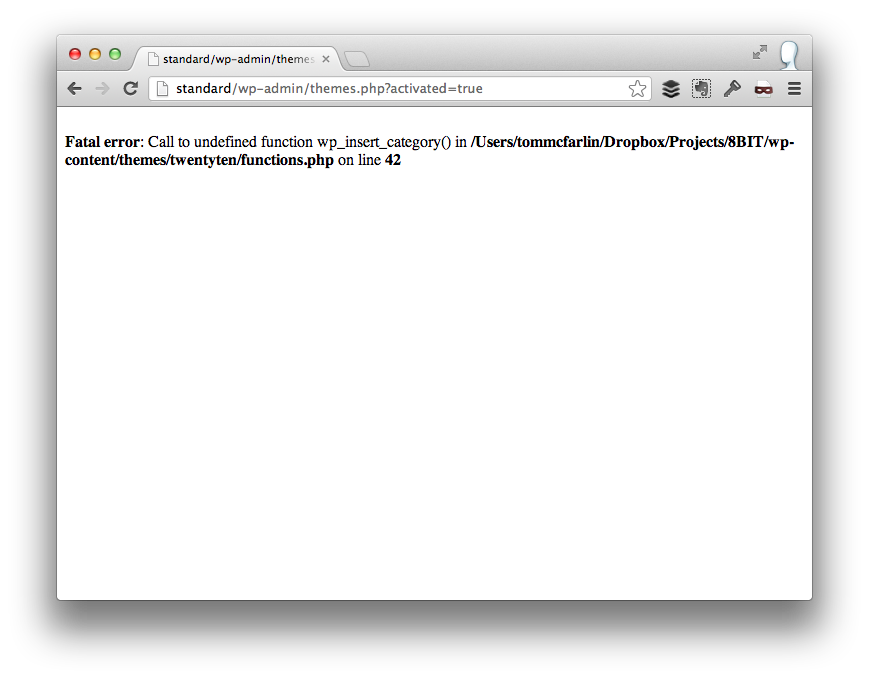
Oops!
Clearly, that isn’t what we wanted. Moar Chasen provided a good explanation as to why this happens in his comment.
That said, there is a second function that WordPress offers: wp_insert_term
.
This particular function doesn’t result in the aforementioned error and I actually prefer it because it’s more flexible with what we’re looking to create.
Programmatically Create Categories in WordPress
Simply put, WordPress taxonomies are ways of classifying data. This means that Categories and Tags are both taxonomies despite the different ways that they are used.
Additionally, if you end up creating your own custom post types and want to create a set of custom taxonomies specifically for said post type, then the WordPress API allows you to do this.
To that end, using the wp_insert_term
function allows us to programmatically create categories, tags, and other custom taxonomies.
For this reason, I’m a fan of using this particular function to create categories rather than anything else that WordPress offers.
To create a category based on the code defined earlier in this post, we’d make the following API call:
function example_insert_category() { wp_insert_term( 'Example Category', 'category', array( 'description' => 'This is an example category created with wp_insert_term.', 'slug' => 'example-category' ) ); } add_action( 'after_setup_theme', 'example_insert_category' );
Refreshing the page will then yield the following results:
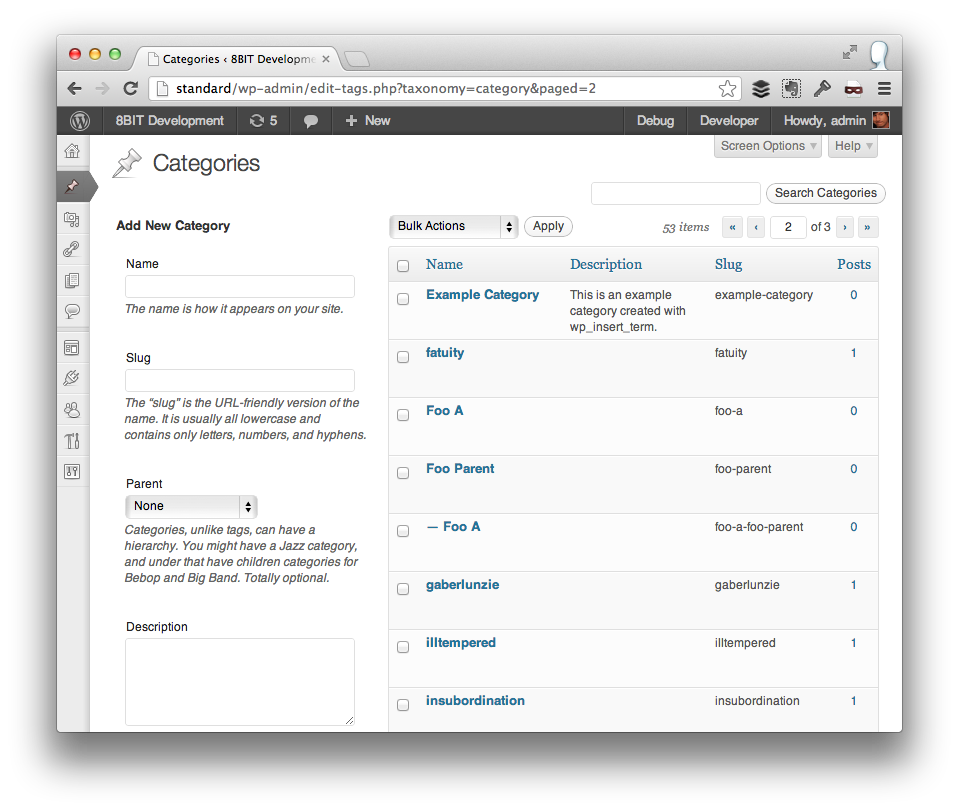
Ah, there we go.
There are a couple of nuances to using this function. Particularly:
Error handling is assigned for the nonexistance of the $taxonomy and $term parameters before inserting. If both the term id and taxonomy exist previously, then an array will be returned that contains the term id and the contents of what is returned. The keys of the array are ‘term_id’ and ‘term_taxonomy_id’ containing numeric values.
It is assumed that the term does not yet exist or the above will apply.
The rest can be reviewed in the Codex article.
At any rate, I’ve found that using wp_insert_term to be much more flexible and clearer when programmatically creating categories (and other taxonomies, obviously) in many of my projects.
Leave a Reply
You must be logged in to post a comment.