One of the things that I find myself trying to do on a regular basis is to streamline how I’m building WordPress-focused functionality. I’ve recently talked about this but thought I’d expand on it a little bit more.
That is, I thought I’d lay out the approach I take when building things such as custom post types, taxonomies, meta boxes, and so on.
Generally, think of this as a strategy that I follow for building out aspects of a project that interfaces directly with WordPress but may require a few components such as:
- classes that register themselves with WordPress through various hooks,
- classes that require calls to certain WordPress APIs
- and classes that require a custom view.
Sure, not every thing that interfaces with WordPress will need all of the above (for example, does a custom post type need a view? No. But a meta box does.)
Organizing WordPress Types
With that said, I’m going to take a more involved example such as a meta box and then break down a way in which I think it can be implemented. I’ll note the things I think are necessary and the things that are optional.
And, as I said, I’m using a meta box as an example because I have a previous reference and it involves the most amount of work whereas something else such as a custom taxonomy may not require all (just a subset) of the pieces.
With that said, let me lay out my approach.
We Need Subscribers
I’ve talked about this particular pattern enough to a point where I’m simply going to link to a definition of it. If you’re reading this page, you’re likely well-aware of the various hooks and how to use them to in WordPress.
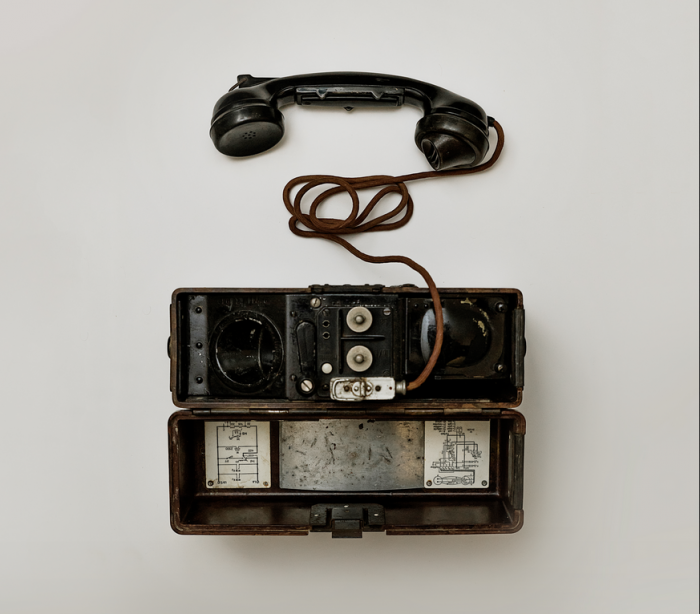
Photo by Alexander Andrews on Unsplash
But the reason I want to mention it is because rather than thinking about hooking up a function to fire whenever something happens, I want you to think of an object that subscribes to an event when it occurs.
This means that we’ll need a type of subscriber class.
WordPress API Classes
Secondly, we need classes that are responsible for interfacing directly with WordPress. These are the classes that call to the WordPress API and register whatever it is they are responsible for doing.
That is, perhaps they are going to define a custom post type or perhaps, as stated, they are going to define a meta box.
Defining Views
Finally, it’s important to note that for some custom functionality for the WordPress administration area (or even public-facing areas), you may want to include a view or a template or a partial (I generally just refer to them as views) that will work to represent the data for a meta box.
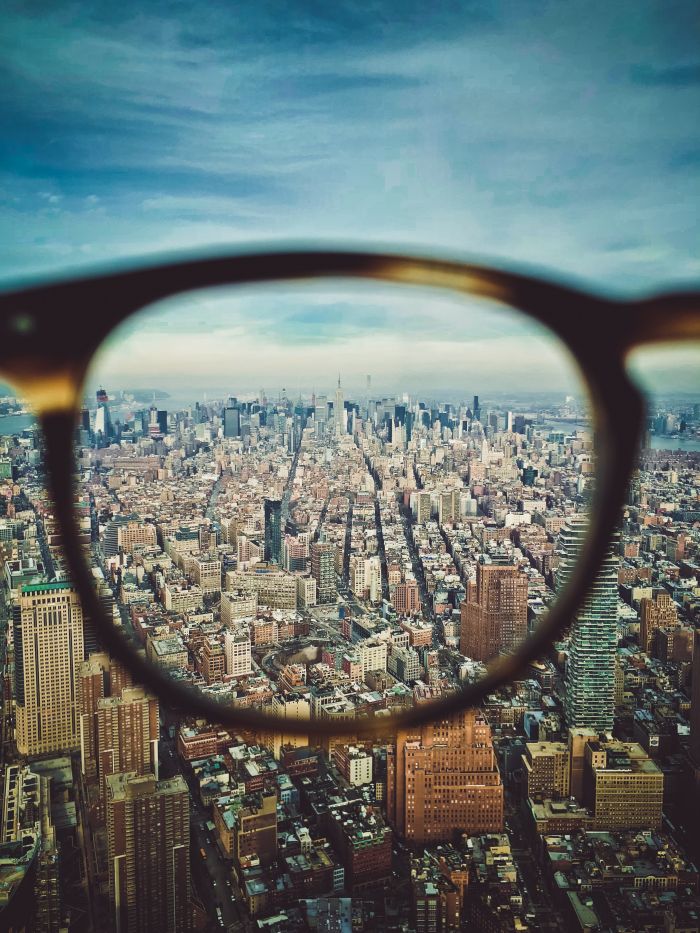
Photo by Saketh Garuda on Unsplash
Sometimes this will simply be informative. Sometimes, this will require that it posts back to the server and serializes the data. Though I think talking about the latter would be really beneficial, it’s outside of the current scope of this post.
Perhaps in a future post.
Organizing Classes
What all of that said, what would it look like to lay all of this out? At the very least, we’re looking at:
- a subscriber,
- a WordPress-type,
- a view
And, at most, you may be interested in defining interfaces or abstract classes to help enforce a contract among the various WordPress types. This is also a healthy object-oriented principle that I’ll talk about in a future post.
For now, though, let’s talk about how to set up each of these.
The Subscriber
Simply put, the subscriber is responsible for listening to whenever WordPress raises an event (publishes an event). And when it notices that it does, it fires off a function that’s hooked to it.
This is generally defined in the registry pattern. If you’ve not read that post, I recommend it, but setting up the code for it is quite easy:
From there, whenever the event is raised, the function will fire. Here’s the thing though: The function has to be part of a certain class. Thus, the need for the WordPress-type
The WordPress-Type
I like to consider the types of things that interface with WordPress as WordPress-types (much like our programming languages have native types such as strings and integers). WordPress has taxonomies, meta boxes, menus, and so on.
For our subscriber to proper work, it needs to be made aware of our WordPress type. In keeping consistent with the meta box example, here’s what it may look like:
Then we need to make sure the registry is aware of this class.
The View
Finally, for a meta box, we need to make sure that there’s a view that will at least display information. Serializing information and then updating the view for the user is a bit of a different beast.
But what might a view look like? Easy:
It’s just basic markup that renders information to the user.
Tying it All Together
Whenever I put all of this together, I usually have a plugin class that gets it all started. If a project is large, there may be more than one, but in this case, I think it’s okay to show what it looks like using a single class.
So, first, the main plugin class looks like this:
And the bootstrap for the plugin looks like this:
And, from there, everything else is set into motion.
What About More Advanced Functionality?
I raise this question because I’ve already talked a bit about this earlier in the post. Namely, I talked about:
- the idea of posting data back to the server (and likely reading it again),
- and I’ve talked about the use of interfaces.
These are both things that I think are worth exploring in more detail. But before doing that, laying the foundation for how I organize this information is it’s built especially given that it’s build on previous posts like the Registry Pattern and organizing WordPress-centric classes via meta boxes, as well.