At some point, anyone building a theme, plugin, or even just working with WordPress has seen the Fatal Error: Memory Exhausted message. It typically reads something like this:
Fatal error: Allowed memory size is 268435456 bytes exhausted (tried to allocated 29596635 bytes) in …/wp-includes/wp-db.php on line 885.
Yes, your message may be a little different, but the point is the same: You see a fatal error, it has something to do with the amount of memory allowed, how much was attempted to be allocated, and what file threw the error.
In my opinion, one of the big problems with errors like this is that it’s far too easy to Google for a quick solution to fix the problem rather than truly understand the problem.
Sure, I understand we’ve got stuff to do and work to get done, but understanding what the problem may be is important to helping us become better developers, and, who knows, we may uncover a bug in a piece of open source software.
In this case, it’s not the latter, but here’s a good way to go about understanding the above error.
WordPress Fatal Error Memory Exhausted
Using the above message as an example, we’re told what the allowed memory size is: 268435456 bytes. But no one deals in bytes anymore, right?
We’re much more comfortable with megabytes, gigabytes, and even terabytes (though if you have that kind of memory and are seeing this error, that’s a whole other situation :)).
Bytes To Megabytes
So the first thing to do is to convert bytes to megabytes. Google makes this really easy: In the search field simply enter:
268435456 bytes to megabytes
And it’ll return a result for you:
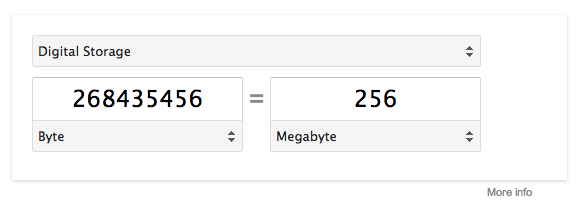
This equates to 256MB
Kind of neat, right? But what do we do with this information?
First, we need to understand that we have a limit of 256MB of memory that’s available to be used by PHP and WordPress and that it was insufficient for the operation.
Honestly, this tends to be more of a limitation of PHP, but I bring up WordPress because part of the solution may involve defining a new constant if you’re using WordPress. But more on that in a moment.
Adjusting PHP
Knowing that PHP has a memory limit and knowing that you have some control over this using WordPress, there are normally two things that I recommend others do in order to make sure that they solve the problem in all the right places.
But first, you need to know what version of PHP you’re using. If you’re running this on a local machine, then you’re likely able to find this within your web server’s control panel:
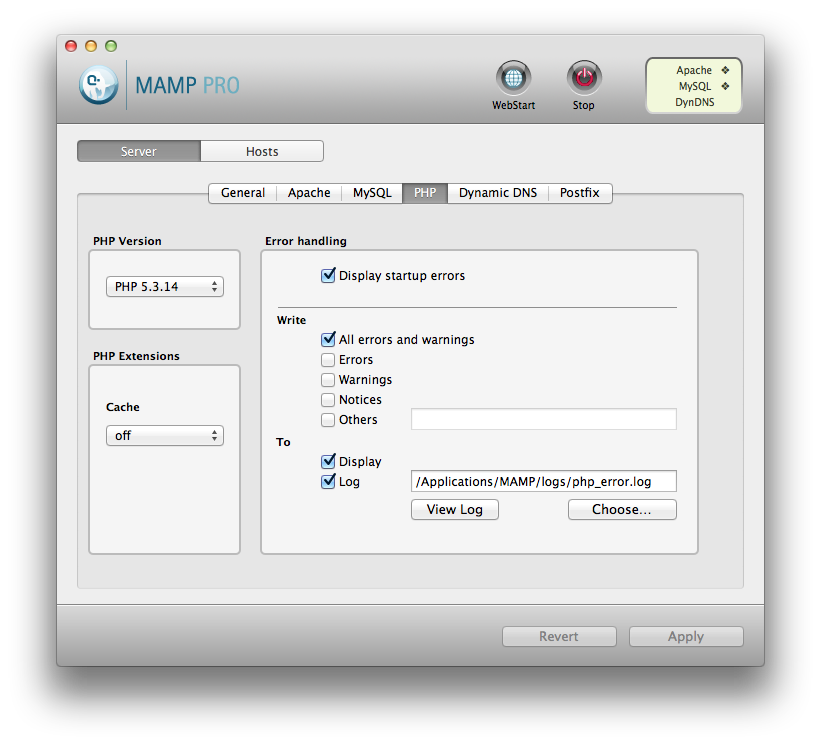
I’m running PHP 5.3.14
Otherwise, your web host should have this prominently displayed somewhere in the dashboard of your hosting account.
This lets us know which PHP configuration file to edit. Specifically, you need to locate the `php.ini` file that’s relevant to your version of PHP.
If you only have one version installed, easy; otherwise, you’ll need to locate the `php.ini` file for the specific version of PHP that you’re running.
Increase The Memory Limit
Once you’ve located the `php.ini` file, look for the `memory_limit` directive:
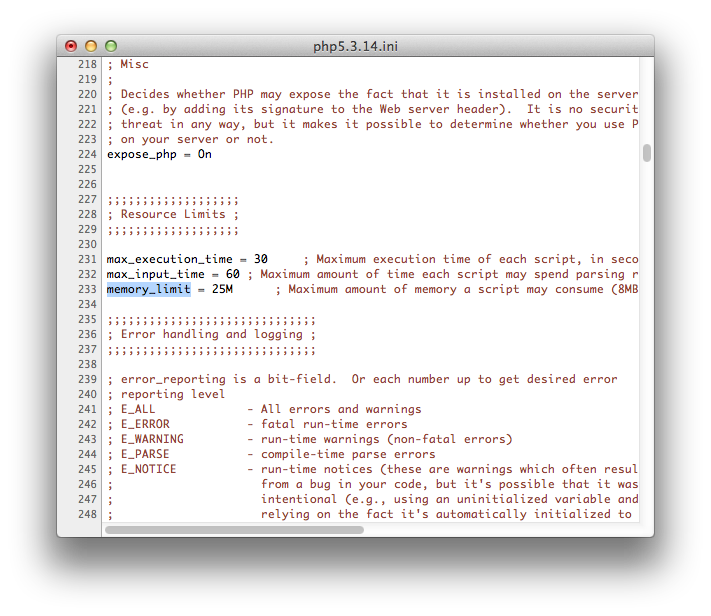
The PHP memory_limit Directive
In the shot above, you can see that the memory limit is set at 25 megabytes which is clearly less than what we need. To that end, you should be able to increase it to, say, `128M`, restart the server and be good to go.
What If WordPress Still Complains?
In that case, then you may need to make one additional change to your `wp-config.php` file. There are two constants that we can define:
- `WP_MEMORY_LIMIT`
- `WP_MAX_MEMORY_LIMIT`
You can read more about both of these values in details in the Codex, but the gist of it is that I’ve had more success defining both values that simply editing `WP_MEMORY_LIMIT`.
I mention this because there is a lot of advice that advises others to define the `WP_MEMORY_LIMIT`. I’m certainly not saying that’s wrong – I’m just saying that I’ve had better results when both have been defined.
So, just as with the `php.ini` file, add the following values to `wp-config.php`:
define('WP_MEMORY_LIMIT', '128M'); define('WP_MAX_MEMORY_LIMIT', '128M');
And that should resolve the issue.
A Word About Memory Allocation
One thing I do want to mention is that although a simple solution may be to simply vastly increase the available memory, this isn’t necessarily the first thing that you should do.
In fact, this is normally the last thing I do.
Often times, this particular issue is a result of a block of code, a procedure, or some algorithm that may be better optimized than how it currently stands when the error is thrown.
So to that end, double-check your code first – use some profiling tools or debugging tools to make sure you aren’t doing anything that’s inefficient. If not, then adjust your memory.
Leave a Reply
You must be logged in to post a comment.