Yesterday, I started a series in which I’m planning to layout to how to use some basic object-oriented practices to work with the WordPress Settings API.
Though I’ve covered the API in-depth before, I’ve found that there are ways we can further improve a project’s architecture if we stick to some guiding object-oriented principles. But in order to do that, it’s important to understand certain things such as classes, interfaces, and functions that are public
, protected
, and private
.
For many, these are well-known concepts, but for others who are just jumping into the world of object-oriented programming (let alone WordPress), it can be a lot to take in and even more daunting to ask for help.
So I’m planning to take this post by post (or step by step or what have you) in order to make sure I cover as much ground as possible without overloading anyone. Oh, and questions welcome.
The WordPress Settings API
As mentioned in the previous post, there are three things that must be done in order to work with the Settings API. You’ll need to register
:
- The section
- The setting
- The field
And once that’s done, you’ll need to define a way to not only display
all of the information related to your your setting, but you’ll also need a way to sanitize
the information to protect it from the database.
All of that will be covered in future posts. Today, we’re primarily focused on understand interfaces and understanding how to construct our first interface.
A Short Primer On Interfaces
According to Wikipedia, a class interface is:
In object-oriented languages, the term interface is often used to define an abstract type that contains no data or code, but defines behaviors as method signatures. A class having code and data for all the methods corresponding to that interface is said to implement that interface
In short, class interfaces define the methods that any class that implements that inferface must implement the methods.
For example, let’s say that you have an interface for a 80’s device called a Stereo
with method signatures for stop
, play
, rewind
, fast forward
, and pause
. This methods that any manufacturer who wants to make a Stereo
must provide functionality for methods named like that.
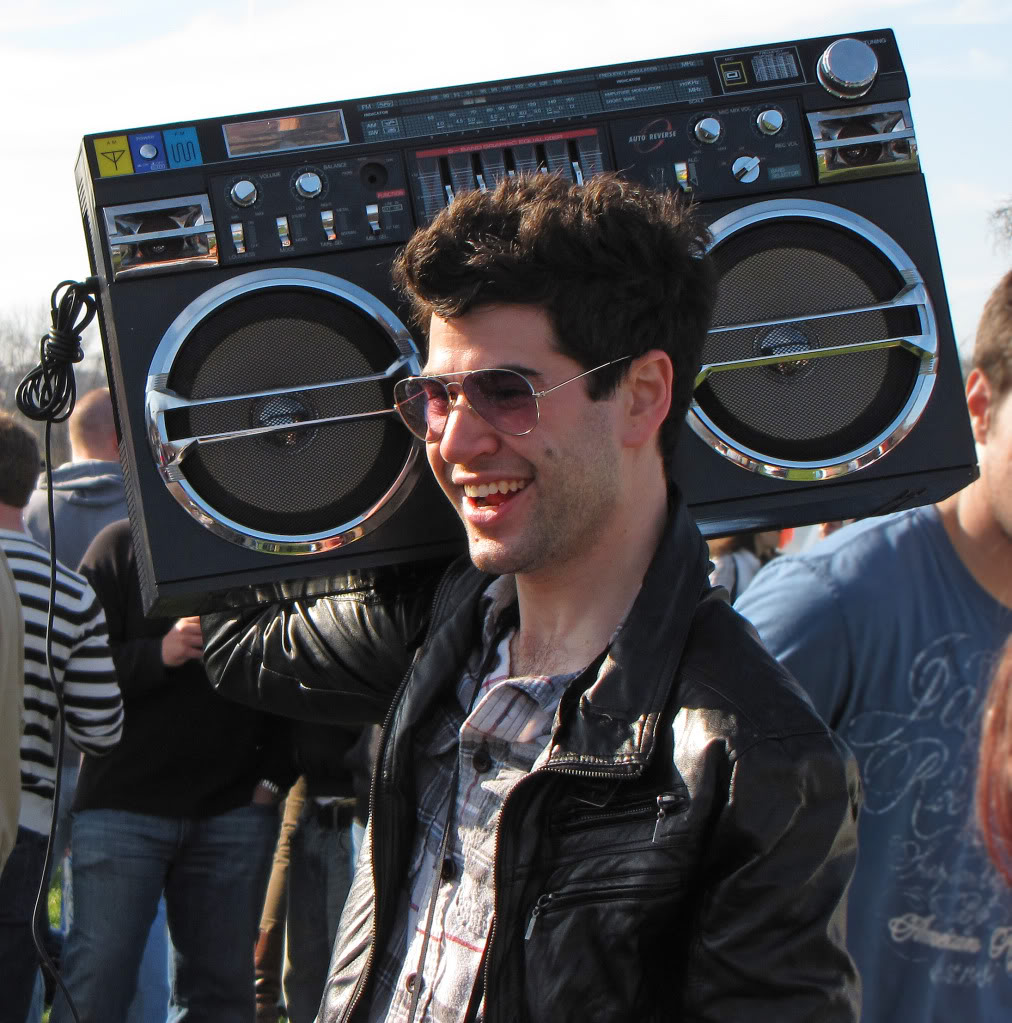
This probably one of you rocking out back in the day.
Make sense? Let’s see it in action.
An Interface For The Settings API
Given what we have above, a that implements the Settings API must do the following things:
- `register` sections, fields, and settings
- `display` the information to the user
- `sanitize` the information when the options are saved
So what would an interface in PHP look like? Perhaps something like this:
For those who are unfamiliar with interfaces, you’ll likely notice two things:
- The file is declared with the `interface` keyword (rather than `class` keyword)
- The functions have no definition
Recall that this is because an interface responsible for providing the methods that implementing classes should define. You can instantiate an interface. The method signatures don’t do any work.
But what does that even mean?
In the next part of this series, I’ll take a look at what it’s like to actually implement the interface by defining a simple setting and then rendering it to the display.
In the meantime, feel free to ask any questions that you have about the code linked above.
Leave a Reply
You must be logged in to post a comment.