One of the things that programmers often talk about is the desire to break programs into smaller components, or functions, so that it makes them easier to trace, easier to read, and easier to debug.
But it’s not all that uncommon to see monolithic functions with a lot of code comments to help explain what’s going on in the program.
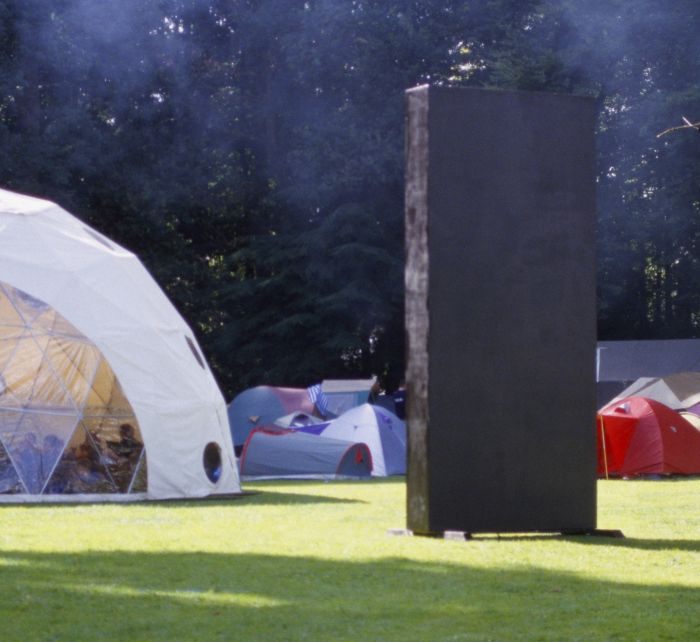
A monolith ala 2001: A Space Odyssey.
I’m not knocking this, really, because I don’t know the constraints under which a programmer was working. That is:
- What was the budget s/he had when building the program?
- How much time was given to complete the project?
- Were there many people working on the project?
- Was the programmer given time to write the code so they could unit test it, refactor it, or merely make it easier to read?
In short, there’s a lot of reason – I believe – that we can read “bad code,” and it doesn’t always have to be the fault of the programmer (that’s just the most natural thing we have to throw out when we read something we dislike).
Does this mean, though, that we shouldn’t strive to refactor or write code in such a way that makes it easier to understand? Of course not. Assuming we have the time to do so, how might we do it?
Break Programs Into Smaller Components
When it comes to writing about a topic like this, especially in an economy that’s as active as eCommerce in WordPress, it can be a challenge.
“Let’s Get Specific, Bob.”
That is, I can write about it at a very detailed level using a suite of plugins, looking at the data, dissecting queries, and showing how to do it. Or I can write it about it at a slightly higher level with the ultimate goal being to show how to break programs into smaller components.
Because there are so many ways the former can be achieved, I’m opting for the latter. That is, this won’t necessarily be using any specific plugins are direct queries. It will, however, be using high-level examples to help you walk through what could be a series of queries and loops and breaking them into smaller functions.
A Generic Example
For example, let’s say that I’m working on a feature of a WordPress plugin that’s ultimate purpose to is to retrieve all of the various payment methods a user has stored and that’s related to their account.
The challenge is that this information is scattered across multiple database tables (because of various plugins that are used) so there are some queries that must be executed and then retrieved.
The steps for making such queries might go something like this:
- get the current user’s customer ID,
- get all of the order ID numbers for the customer
- determine what payment methods were used for each order
- retrieve the said payment methods and then send the information make to the customer
Depending on how the database is set up, depending on your level of SQL prowess, and depending on how the various plugins for handling all of the above data work in tandem, it may be easy to write one large query to retrieve this information.
But if you’ve worked with eCommerce in WordPress and various plugins, you know it’s not always that easy.
Instead, you’re looking at something such as:
- we need to get a customer’s profile from the user metadata,
- we need to find all of the orders the user has made, and this can often be associated with the post or the post metadata table,
- the payment methods can very likely be stored in their table associated with the user through some type of token,
- the token above sits in a table and is related to a given piece of information in another table from which you have to then deduce by looking at the data that exists throughout the database.
Ultimately, you have to create a set of queries only by first understanding how to query for the data for which you’re looking. That’s challenging enough as it is. But when you get to do that, let’s say that you’re writing your queries sequentially and then using the results of each to get to your desired output.
This can result in something like this:
But it doesn’t have to be this way.
First, these are all independent queries with independent sets of results even though they have to be used in tandem. This means we can break them apart and evaluate the results of each before moving forward with the next step.
Furthermore, it allows us to write smaller, more cohesive functions. Even though they may depend on one another, we can set up each function to accept an argument (or set of arguments from which we can retrieve all of the information.
Perhaps the final result may look something like this:
Of course, I can’t show any actual SQL – well, at least not everywhere – because I don’t know the general set up nor do I know exactly what plugins or schemas it is with which you’re working.
But that’s never been the point of this post.
Instead, the ultimate point that I’m trying to convey is this: Even though we may be working under highly limited constraints, we can still break programs into smaller components that help us describe what’s happening, understand how it’s done, and then sending data back and forth between various functions and to and from the user.