Spend enough time working in WordPress, and you find that a lot of data is often held in arrays (and multidimensional arrays, at that). I’m not arguing whether or not this is a bad thing or a good thing; it’s more of an intermediary step between grabbing something from the database and then rendering it on the page.
As you venture into the world of WordPress development, though, and you aren’t necessarily sure how you want to manipulate some of that data before rendering it, saving it, or working with it, you may ask yourself the following question:
How do I read the associative array values in WordPress?
And if you have experience in PHP, this isn’t something that’s terribly difficult. But if you’re working with an existing structure or trying to extend or manipulate something that already exists,
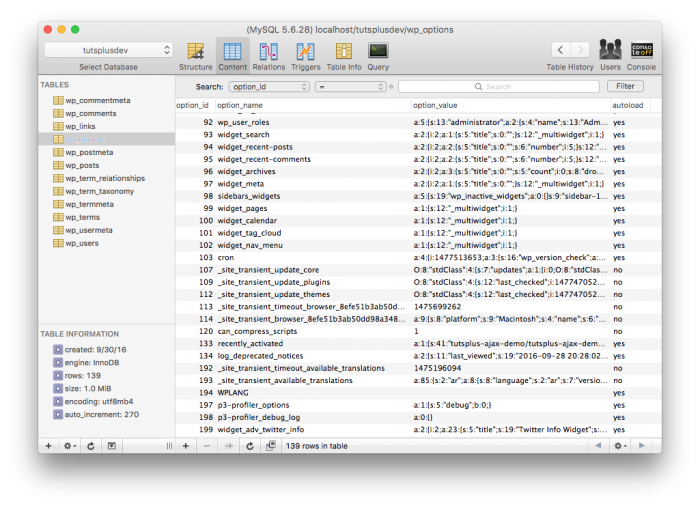
Look at all the serialized options.
But if you’re still learning the ropes and you start trekking down the PHP manual, you’re likely to find something like array_values or array_intersect_key or something like that only to find out that you’re more confused than when you’ve started.
So what are you to do?
Associative Array Values in WordPress
First, note that associative array values in WordPress aren’t necessarily different than associative array values in PHP (or in any other language; however, PHP uses dynamic array sizes).
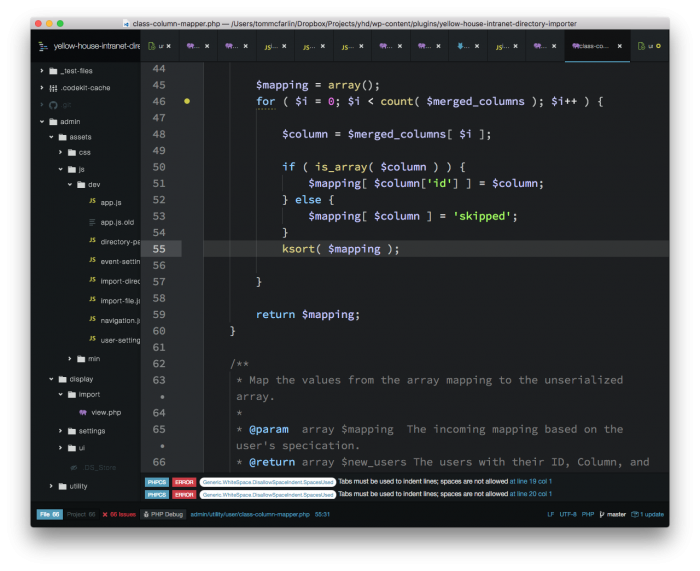
Yet another case of working with arrays.
But I focus on WordPress. Thus this posts contextualizes the example as such.
1. Don’t Save The Changes (Maybe)
If you find yourself working with an array – or any data structure for that matter – and you’re looking to change up the structure of it, then it’s important to remember the format in which it came.
That is, if you’re looking to make changes to the data in the structure and write it back to the database in the same format, then this may not necessarily be the post you’re looking to follow.
2. So What’s The Purpose?
Ultimately, the purpose of reading values from a multidimensional array can vary.
One of the cases in which I’ve had to do this recently is when working with an intermediate step that exists between reading temporary data provided by the user, formatting it in a cleaner data structure, and saving that result to the database.
3. How To Do It
With that said, there’s really a two-step process I tend to follow when doing this (and I break it down like this in case you want to place it in a small class, small function, or a small utility you can easily use).
- Change the original array into a one-dimensional array,
- Work with the one-dimensional array to deal with the values.
Granted, you can always make the argument “yeah but what if the array is .” If that’s really the case, what are you working on? But seriously, the following steps still apply but just divide and conquer.
One Dimension
First, take the multidimensional array and change it to a one-dimensional array:
Now you’ve got one large array with a unique key and, thus, a unique value.
Read The Values
From here, you can do whatever you want with the values:
Perhaps you want to filter out some of the information, or you want to do some other type of processing on the values. Whatever the case, you’ve got the values easily accessible to do whatever it is that you need.
Don’t Dismiss This
And I know, this might seem trivial or simple. But when you’re working with multi-dimensional arrays, and you’re having to conceptually map all of the data like this in your head while also traversing the data in that format, you may end up getting confused easier than you thought possible.
So don’t dismiss a problem (or solution) just because it seems easy at first glance. Everything seems easy after we know how it’s done, doesn’t it?
Remember, You’re Not Done
It’s worth reiterating that when you’re working with associative array values in WordPress, you need to be mindful of if you’re writing the information back to the database.
Because if you’ve changed the original data structure, you’re writing back different data. The code above has more to do with working with information in the intermediary and processing it as you see fit then doing whatever needs to be done to serialize it.
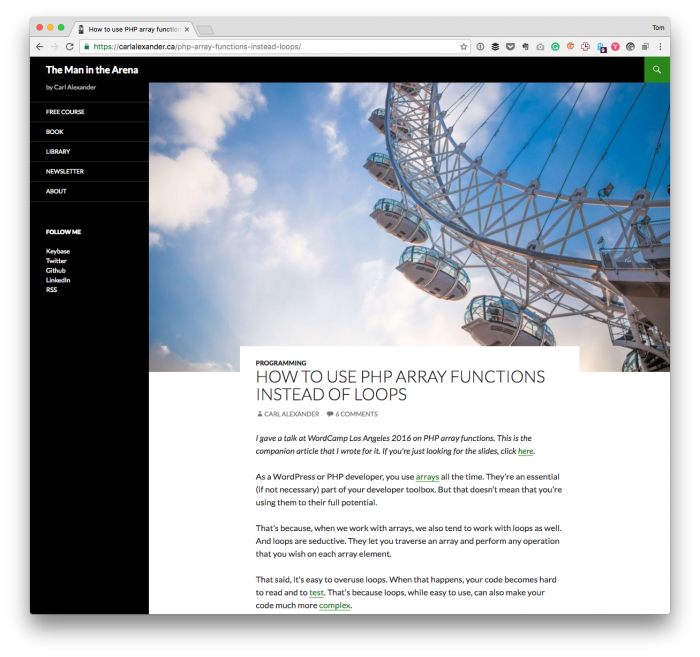
How to Use PHP Array Functions Instead of Loops
Finally, if you really want to get into the trenches with PHP’s arrays, then I recommend reviewing Carl Alexander’s excellent piece (that’s part of a talk given at a WordCamp earlier this year) How To Use PHP Array Functions Instead of Loops.
Leave a Reply
You must be logged in to post a comment.