When it comes to writing plugins – specifically those that are object-oriented in nature – many of us do so because we’re obviously fans of writing object-oriented code.
Personally, I’m a fan of it because it helps to separate the responsibilities and concerns of a requirement into its own logical unit.
Sure, this can be done with several function files as well, but I come from an object-oriented background, so when I approach a problem, I automatically begin thinking in terms of classes and their relationship to one another.
And I know that other developers do the same.
The thing is, I think some of us – myself included – have gotten lazy or we only half-bake our object-oriented-based plugins. That is to say that we may be using classes in writing our plugins, but we don’t do such a good job of taking advantage of other object-oriented principles and features.
An Object-Oriented WordPress Plugin, But Not Really
Here’s the thing: It’s relatively common to see a class-based plugin and then assume it’s object-oriented. But simply using a single class does not truly make it object-oriented.
Instead, I’d say that you’re using an object-oriented feature, but you’re not truly object-oriented programming.
For example, a lot of plugins will use a single class to do their work. A high-level diagram would look something like this:
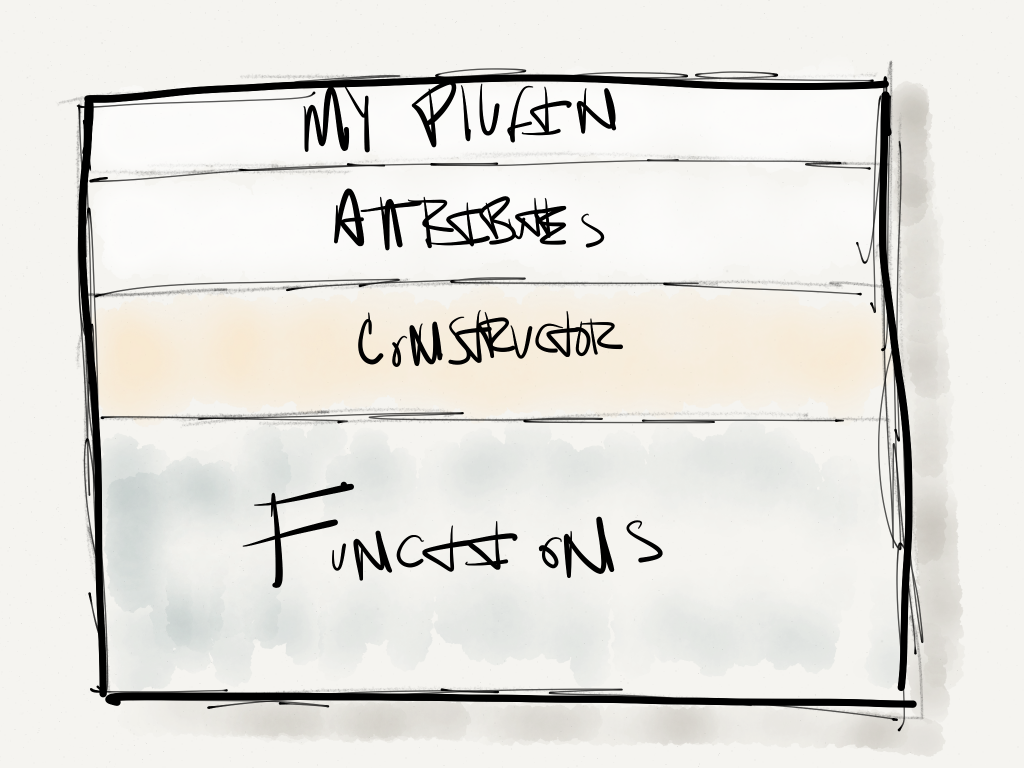
The god-class of a WordPress Plugin
Basically, you have the follow setup for your plugin:
- The class name
- Any private attributes that may be used throughout the class
- The constructor were hooks and other initialization code is setup
- Then a huge area dedicated to functions
This isn’t necessarily bad, but if you’re aiming for truly object-oriented code, there’s likely a need for refactoring.
If you have a single class that’s doing all of the work, then it likely has multiple responsibilities which decreases its identity. Quite simply, you have a god-class – or a god-object – and this is an antipattern.
It’s easy to get away with doing this. Look at a lot of my source code, and you’ll see that I’m doing this same thing. I hate it, but I rationalize doing it because it’s faster and the plugin is free (I’m careful not to do this in commissioned plugins, but that’s a topic for another post).
Have Some Class: Break Up Your Work!
Punny, right?
Anyway, in true object-oriented programming, a given class would have a single-purpose and the attributes and methods would be related to that single purpose.
This means that what once was a god-class would be broken up into smaller, more logical units of code. Yes, this creates more classes, but more classes isn’t necessarily a bad thing.
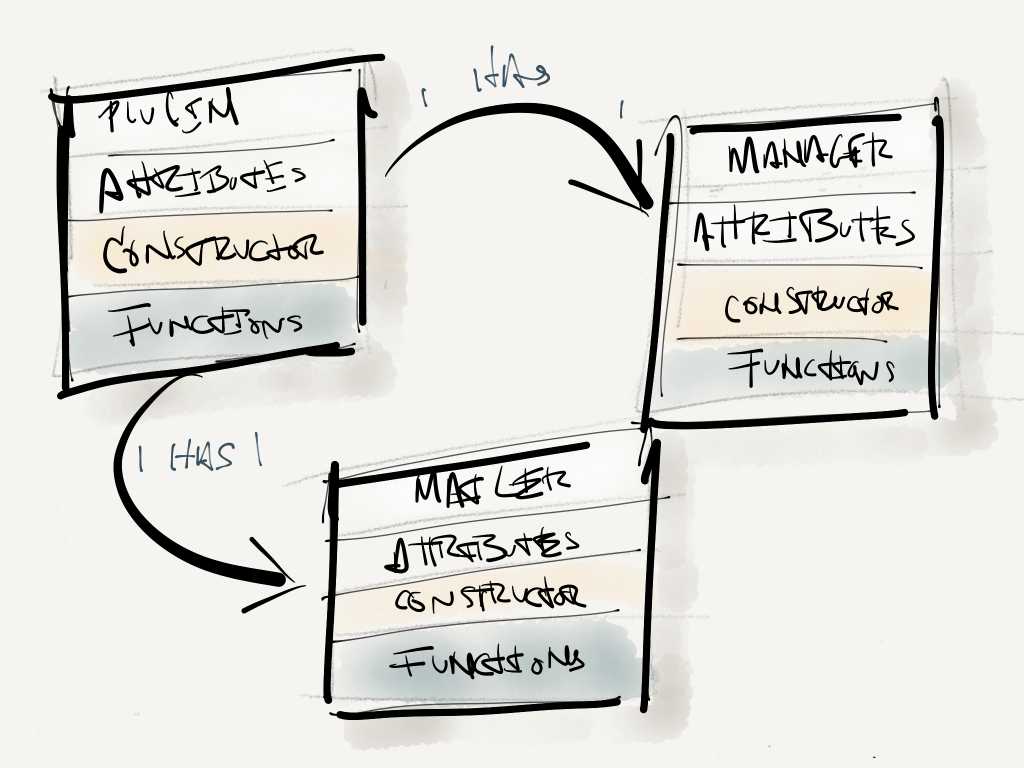
A more cohesive plugins
In this example, you have three classes each with its own set of attributes, initialize code, and functions. Additionally, each class serves a single purpose and not all of them are aware of one another.
In fact:
- There’s a core plugin file that would ideally be instantiated when the plugin is called
- It has a reference to a Mailer class which is responsible for mail operations
- And it has a Manager class which could be responsible for, say, creating, updated, and removing users from the system that are created by the plugin
On top of the that, the main plain class has a reference to each of the other classes, but the Mailer isn’t aware of the Manager. Why should it be? In this case, the core plugin class needs only to send data to and from each class.
Ultimately, this makes for more highly specialized, focused code. It gives each class a stronger level of identity, and it makes maintenance a heck of a lot easier.
Rewrite All The Code! (Or Not)
This is one of those things that is actually easier to do from the start of a project rather than refactoring an existing project.
This isn’t to say that it can’t be done, but the amount of time required and the amount of testing required to make sure that nothing is broken could be far more substantial that you – or a team – is willing to invest.
Regardless of what route you choose, I think there’s room for improvement in how we’re all writing our object-oriented plugins. Again, I’m guilty of this just as much as the next person so I’m writing more to myself than anyone else.
But it’s nice to get it out in the open so I at least know I’ll be held accountable if I release something that doesn’t actually practice what I’m preaching, you know?
Leave a Reply
You must be logged in to post a comment.