When I first started working on WordPress plugin development, I used to obsess over the right hook to initialize WordPress plugins. That is, I thought there was one hook that would sit above all other hooks and prevent me from needing to go looking through the Codex or other source code to find the right one.
But that’s not the case.
To be clear, this isn’t to say that there aren’t some strategies and some hooks that work better than others in many, many cases, but there are times where whatever you’re used to using isn’t going to work. This depends on how you’re structuring your plugin, and I’ll talk more about that in a moment, but there’s isn’t one hook to rule them all when you want to initialize your WordPress plugins.
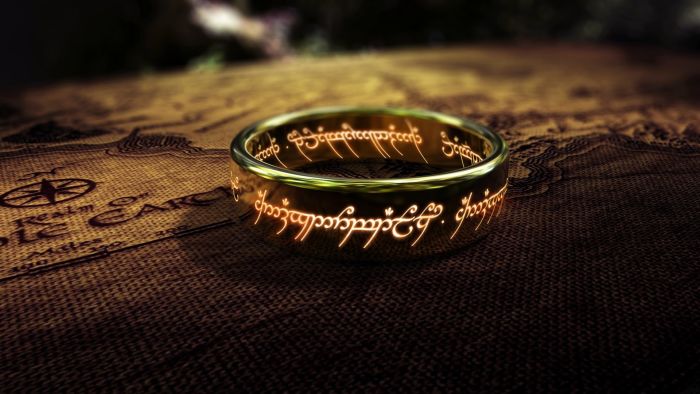
By now, you likely know the whole deal about the one ring, right?
Instead, you’ve got to find the one that suits your needs best for the functionality you’re introducing.
Initialize WordPress Plugins
For this post, I’m assuming that you’re relatively familiar with what goes into creating a WordPress plugin. Perhaps you’ve even experimented with a few different ways to go about doing it.
By that, I mean you’ve tried:
- Implementing the singleton pattern and starting it in a core plugin file,
- Or maybe you’ve defined a function and called it from a core plugin file,
- Or maybe you’ve even instantiated a class and added it to the `$GLOBALS` collection
Whatever the case, each of these works and each of these has their place (except maybe that last one, but that’s another post), but there are times where even the other two aren’t enough.
Take, for example, the case where you have a plugin that has to check a user’s permissions, and you have to check the page they’re on before firing off certain functionality.
In this case, you’re likely using the functions:
And these are just two examples, but if you look at the documentation for get_current_screen
, you’ll find the following sentence:
The function returns null if called from the admin_init hook. It should be OK to use in a later hook.
This doesn’t even include current_user_can
. So what are we supposed to do? Rearchitect the entire plugin in hopes that we’re able to cobble together something that works?
No way!
We continue to take advantage of the hook system. We define our functionality, look up the order of the hooks as they fire, and then appropriately prioritize when our function should fire.
Assume for the example in my Acme_Class
I have a function that calls get_current_screen
. So I hop over to the Codex page to see the order in which the actions fire, and then I notice current_screen fires right after that.
For my code, I want it to relatively late in the process, so I’ll give it a priority of 99:
And here, I’ve taken a function that I originally wanted to use but wasn’t able to do so because it required other functions fire first. So, through the use of documentation, I found the next best hook to use, applied an appropriate priority, and the plugin works as expected.
It didn’t require a rewrite. It didn’t require rearchitecting. All it required was a little research, a continued used of the WordPress API, and I was ready to go.
This isn’t to say that things won’t be a little more complicated for your case, but before you start trying to rewrite entire functions, see if you can’t leverage the existing API to get your current approach working.
Odds are, you’ll be able to do so (and you’ll learn something along the way).
Leave a Reply
You must be logged in to post a comment.