Say you’re working on a project in which you want to provide the user the ability to download all of the email addresses from the users that exist in the WordPress installation. Granted, this has a few requirements:
- You only want administrators to do it,
- It needs to be secure via nonce values,
- The responses should be in JSON,
- And you want to use JavaScript to send the file when it’s ready to download.
Each of the above concepts has likely been covered here before, but what if you’re looking to tie them all together? That is, what if you want to make it easy to download user email addresses via JSON within WordPress from, say, an administration menu or a submenu?
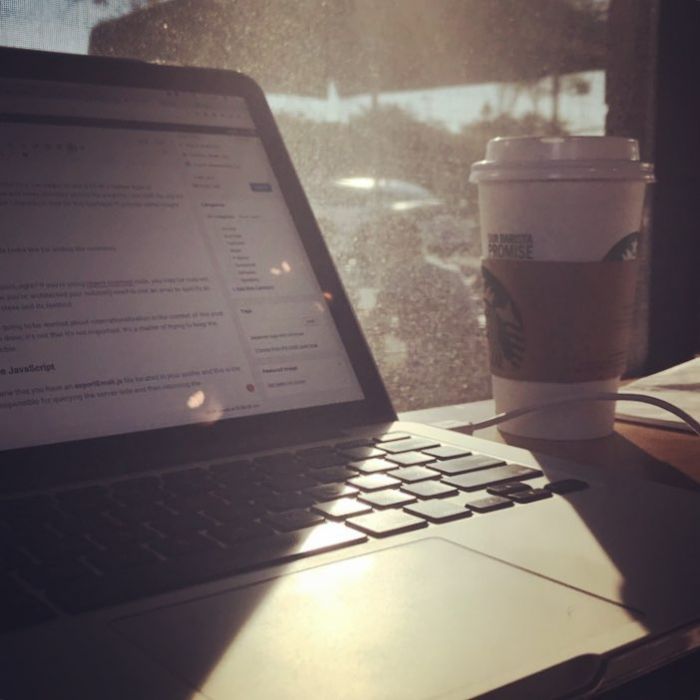
An obligatory shot of where I’m working while writing this post.
Here’s one way to tackle that problem. Note that it does assume you have some knowledge of the above, but I’ll do what I can to cover each of the above.
Download User Email Addresses via JSON
Before getting started, note that I’m setting this up to work whenever a user clicks on a submenu under the Tools menu in WordPress.
How you set up your hooks may vary. I’ve begun to use a bit of a particular type of architecture that’s becoming more and more standard across the projects I use both for myself and that we use at Pressware, but I digress on that for this (perhaps I’ll provide some insight into this later).
Adding a Submenu
Anyway, here’s what the code looks like for adding the submenu.
It’s exactly what you’d expect, right? If you’re using object-oriented code, you may (or may not, again, depending on how you’ve architected your solution) need to use an array to specify an instance of the current class and its method.
Note also that I’m not going to be worried about internationalization in the context of this post. It’s not that it can’t be done; it’s not that it’s not important. It’s a matter of trying to keep the post as lean as possible.
Registering the JavaScript
I’m going to assume that you have an exportEmail.js file located in your profile and this is the file that will be responsible for querying the server-side and returning the file for download.
The first thing that to do is securely register the JavaScript on the server-side using the standard API functions:
Once this is done, you can write the JavaScript that will call the server-side. But this is a two-part step: It includes both JavaScript and then more server-side code.
The Client-Side Calling the Server-Side
Since the basics are in place for calling the server-side, it’s possible to set up the JavaScript now.
First, there’s the click handler:
The initial get call should look something like this:
Notice that it uses the security value we created earlier and then it uses the name of another function, namely getEmailAddresses, that it’s calling on the server-side.
This function is responsible for running a query, grabbing the email addresses, and writing a temporary JSON file to return to the user.
1. Get the Users’ Email Addresses
First, WP_User_Query will make it possible to pull back all of the users for certain criteria.
For the purposes of this example, I’m obviously going light on the arguments.
2. Parse The Email Out of the Results
Next, I’m going to iterate through the results and store the email addresses into an array (that I’ll eventually convert to JSON).
Note there are other ways to do this. This is but one way to do it for a smaller set of data, but if you’re working for something a bit larger, then I recommend an alternative (which is beyond the scope of this post).
Up Next
Obviously, this is just the first part of how to wire all of this up.
In the next post, I’ll cover:
- converting the returned information into JSON,
- writing that file to disk,
- and how to tie the rest of it together using JavaScript.
The final step will include creating an element and then triggering its click event so that the user is presented with the download dialog rather than the actual text in a browser window.