A client-side Ajax protocol is arguably more about parsing the response from the server than it is implementing actual functionality.
This isn’t to say that as the server-side protocol mature, the client-side won’t as well. But, often, what changes on the server is what will dictate change on the client.
By that, I mean that if we’re to introduce a new message in the protocol, then it will be first added to the server-side protocol and then the client-side protocol will need to support it. Rarely will it happen the other way around (at least in the types of projects that are built on WordPress.
With all of that said, how do we support the protocol in JavaScript (or on the client-side)?
Client-Side Ajax Protocol
Working with this type of functionality in JavaScript can usually be broken down into three, very general steps:
- making the request to the server,
- parsing the response from the server,
- taking the appropriate course of action based on the response.
Making Ajax requests with WordPress is straightforward (and assumed so in this article) given what I’ve covered and what’s available in the WordPress Codex.
A simple example of making a request that will communicate with the server (and the protocol outlined yesterday) might look like this:
At this point, we know to expect a response in JSON from the server because that’s how we’ve built the protocol to work. And this is where the parity between the server-side and the client-side needs to exist.
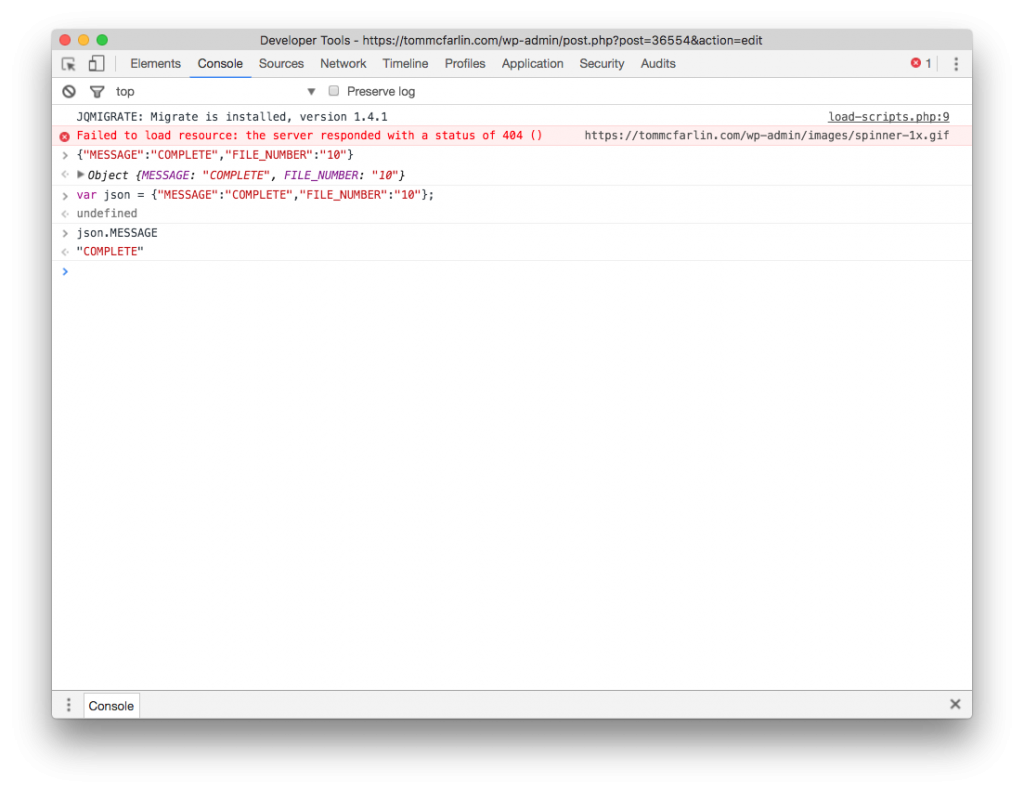
Testing a JSON response in the console.
That is, the client-side needs to be prepared to handle any of the potential messages that the server returns.
In this example, the client should know what file number was sent because it sent it in the first place. This doesn’t mean we shouldn’t check for it, but to keep the code simple, I’m not focusing on it in this post.
A very, very simple way in which this can be achieved is like this:
However, I want to mention a few things about the code above:
- It’s generally better to set up a new JavaScript object (rather than a function) to handle the response.
- Doing this will yield greater testability, portability, and less convoluted code especially as the protocol matures.
- The code above is meant to serve as an example, not as a production-grade stand-in. It’s a demonstration of a concept, not a working library.
The final implementation, tieing it all together, would look like this:
Note that I’m not a big fan of using the switch
statement, but in this case, it demonstrates the point. If the protocol is more advanced, I think using a simple factory and having objects for each of the possible messages is a better architecture.
But that’s far beyond the scope of this series.
How you opt to implement what’s above will depend on your environment and what other libraries you’re using. WordPress uses jQuery and has its API for working with Ajax. Thus, the code above adheres to that using said library.
A General Approach
The general points remain:
- parse the response,
- examine the message (and any payload it may have),
- respond appropriately.
Approaching Ajax functionality across projects will provide you with a formula that works for many WordPress-based projects.
Series Posts
- Protocol Syntax
- The Server-Side Ajax Protocol
- The Client-Side Ajax Protocol
Leave a Reply
You must be logged in to post a comment.