One of the common things that I’ve seen – and personally done – is mismanaging $_POST
data as it comes into the server-side from a form or some type of input element from the front-end. This may be in the case of sending data via Ajax or by doing a standard page refresh.
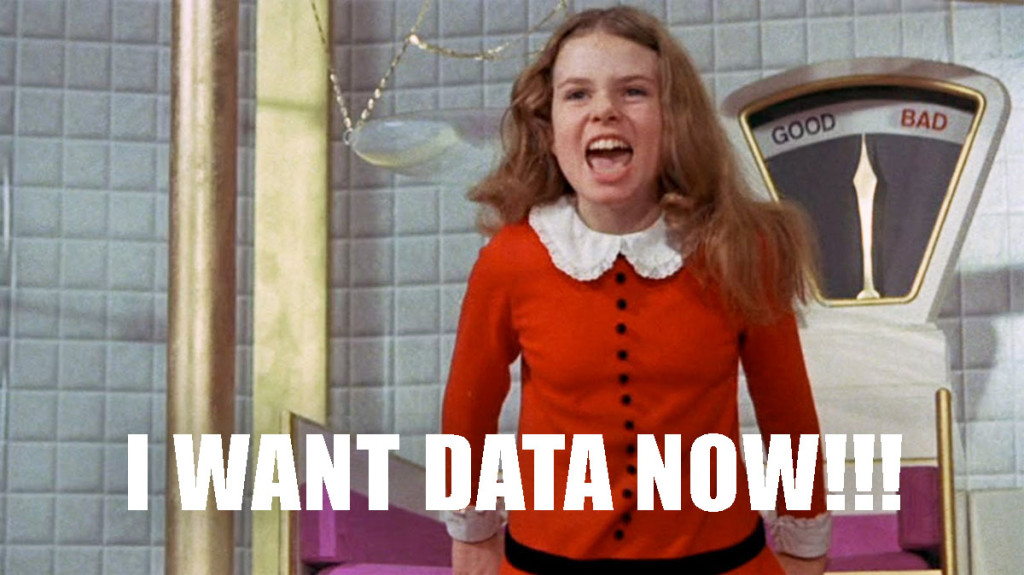
Chill Out, Veruca. We’re getting there.
Whatever the case, whenever you’re dealing with $_POST
data in WordPress and you’re looking to save information into the database, there may be times where you’re interested in saving empty values, and there maybe times where you’re interested in savings values only if they are not empty.
If you’re going after the latter, there are a couple of safe guards you should introduce in your code to make sure that rows are only being written when there’s data to actually be written.
Post Meta Data
In order to talk about this, it’s requires some type of concrete example.
In order to keep the code as focused as possible, I’m not going to be focused on the sanitizing techniques that WordPress and PHP provide. Suffice it say that we need to make sure we’re properly escaping information, stripping any unnecessary HTML, removing any other tags, and verifying any relevant nonce values.
Again, this is being left out of the code in order to make sure we’re focused on the primary problem at hand.
With that said, let’s say that we have a single input element named acme-first-name
and we’re looking to serialize this as post meta data for a given post. Assume that we’re given $post_id
to represent the post, and assume that we’re sanitizing all of the data properly.
Saving Post Meta Data
Saving the information isn’t really that that difficult – in fact, it’s pretty straightforward; however, this is one way of doing so that I’ve seen far too often:
The problem with this is that just because you’re checking to see if the index of an array – here, namely $_POST
– is set (or, rather isset
), doesn’t mean that it holds a value. After all, holding an empty string counts as holding an empty value (because an empty string is not nothing, right? :). And thus, you can actually have empty rows in your database.
One of the most defensive ways that I’ve found to go about doing this is as follows:
Easy enough: trim
the data, verify it’s length is 0, and if it is, then save the information to the database. This will prevent empty rows from being saved.
Getting Post Meta Data
Retrieving the post meta data is as simple as simple as making a call to get_post_meta
, but there’s an important distinction to make as noted in the Codex:
If there is nothing to return the function will return an empty array unless $single has been set to true, in which case an empty string is returned.
Note the last part of the sentence: “in which case an empty string is returned.”
Now, there are times in which you may want to save an empty string and if that’s the case, then the general strategies outlined at the first part of this post don’t really apply; however, we can make for more defensive coding practices if we know what we’re looking for.
Since we’re not going to be saving an empty string, there are a number of ways for which we can check if the post meta data exists, but since the row is only being written if there’s actual data in the `$_POST` array, then we can set the third parameter to `false` and check its length (or check whether or not its `empty`).
Shy of setting up a routine to exhaustively check of the information doesn’t exist in all ways compatible with what the API returns, this allows us to write defensive code both when we’re saving information and when we’re retrieving information.
Beyond the Post
For those who have been working with WordPress for sometime know that meta data can exist for more than just posts – we can introduce it for users, comments, and other models or types of data (depending on how you term the information).
Generally speaking, the same strategies apply. Aim only to save information when you need to save it – it keeps the database clean, and it makes it easier to retrieve and test information when it’s released.
Leave a Reply
You must be logged in to post a comment.