Creating Slack applications can be as time-consuming and complex as writing any other type of application. What if you’re interested in querying the Slack API for your workspace and incorporating the results in a WordPress plugin? Here’s how you can get started.
Ultimately, all we need to make queries to the Web API is an OAuth token and a REST API client to make requests. From there, it’s a matter of programmatically implementing the functionality in your code.
But that’s not at what we’re aiming. Instead, this article is more about learning how to make requests to the Slack API and what’s required to get set up to do so.
Querying the Slack API
To get started, you really only need three things:
- A Slack account (the desktop application is what I use but the web application is fine; we won’t really be using it so much as we need an account to get an API key),
- A Slack application which we can create using tools they’ve provided,
- A REST API client like Insomnia.
And that’s it. So assuming you’ve got a Slack account or you’re able to easily create one, we’ll pick up there.
Create a Basic Slack Application
From the Slack API page, click on the button that says Create an App. When you do so, you’ll be taken to a page that prompts you to choose what kind of app you want to create.
For the purposes of this article, choose From Scratch. The description being:
Use our configuration UI to manually add basic info, scopes, settings, & features to your app.
From there, you’ll need to fill out some basic information about your app. You can choose to give it whatever name you want (at least if this is a demo application 🙂).
Grab the OAuth Token
Next, you can choose how many features and how much functionality you want to enable. When getting this demo together, I opted for Incoming Webhooks, Bots, and Permissions.
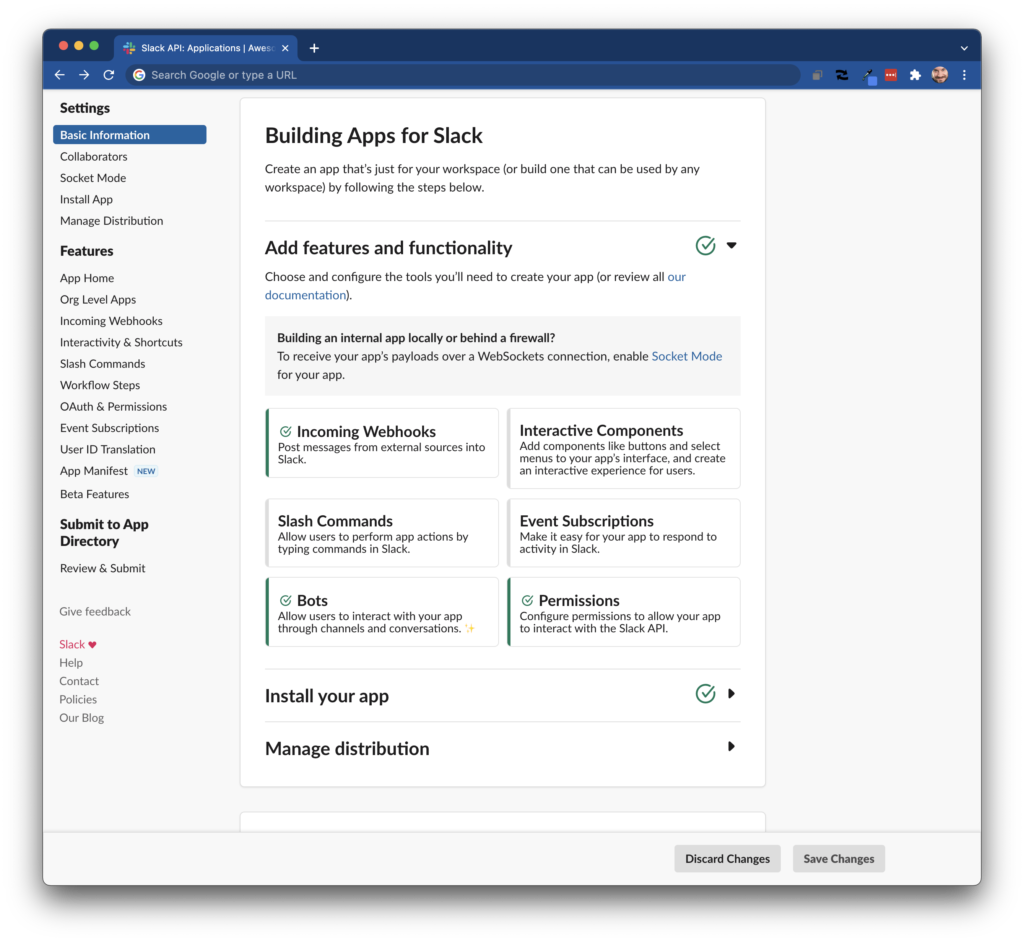
After that, I installed my the app in the workspace in which I was working. This can be a demo workspace you’re using or it can be one in which you already operate. Regardless, the app can be deleted at any time you’re done with it.
Make a Request with Insomnia
Once done, locate the link OAuth & Permissions in the sidebar of the documentation. You should see a section that reads:
OAuth Tokens for Your Workspace
These tokens were automatically generated when you installed the app to your team. You can use these to authenticate your app.
Just under that, you’ll see a text field from which you can copy a token. The token should be in the form of xoxb-...-...-...
. This is the OAuth key that we’ll use to make our requests.
You can view a list of all of the available API methods on this page.
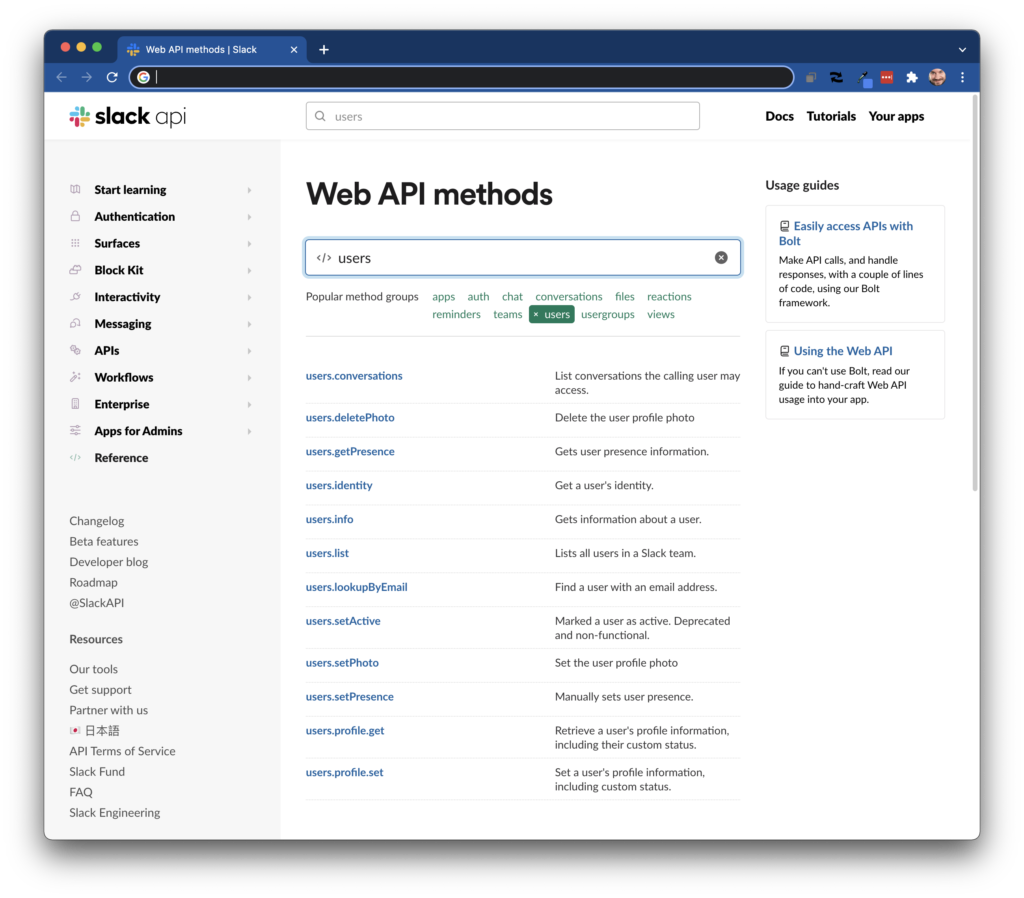
It’s actually possible to test some of these methods in the browser.
Testing in the Browser
Before hoping over into Insomnia to see what we can do, let’s test drive one of the methods in the browser. Remember to make sure you have your OAuth token from earlier.
We’ll test the getPresence
method which will give us some information about whether or not the user specified by a Slack User ID is currently online or not.
First, in Slack, grab the member’s ID by clicking on their avatar then clicking on View Full Profile. If you click on the More text, you should see a field that says Copy member ID and an identifier below it. Click on that to copy the ID to the clipboard.
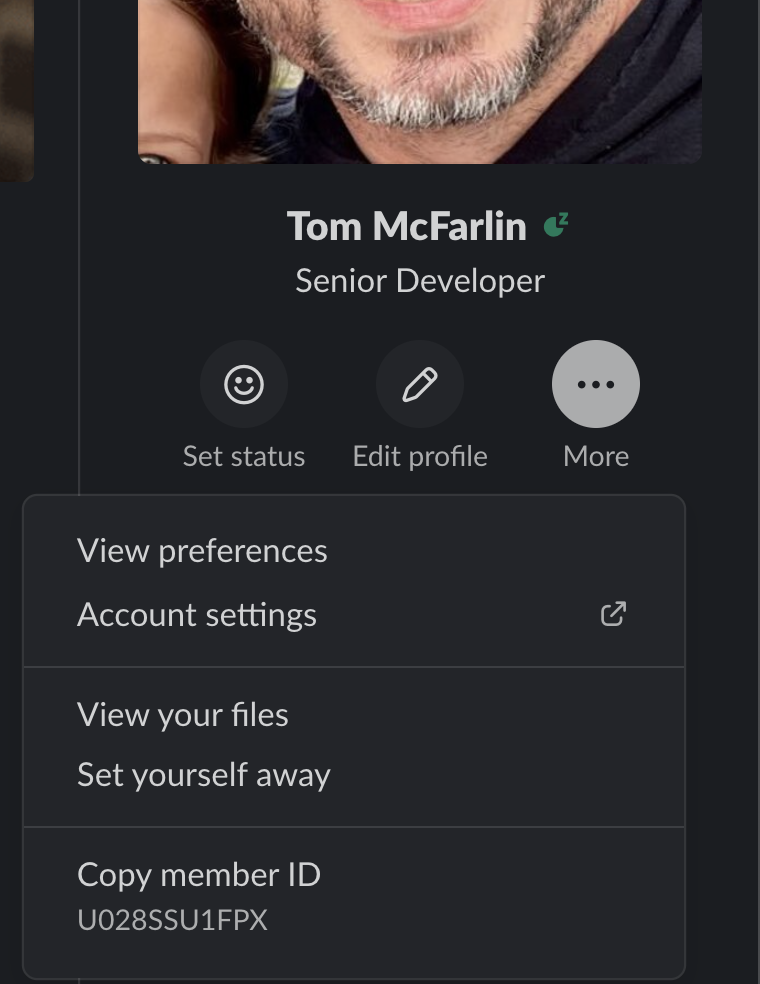
From there, use your token and the ID in the tester and fill out the form like this:
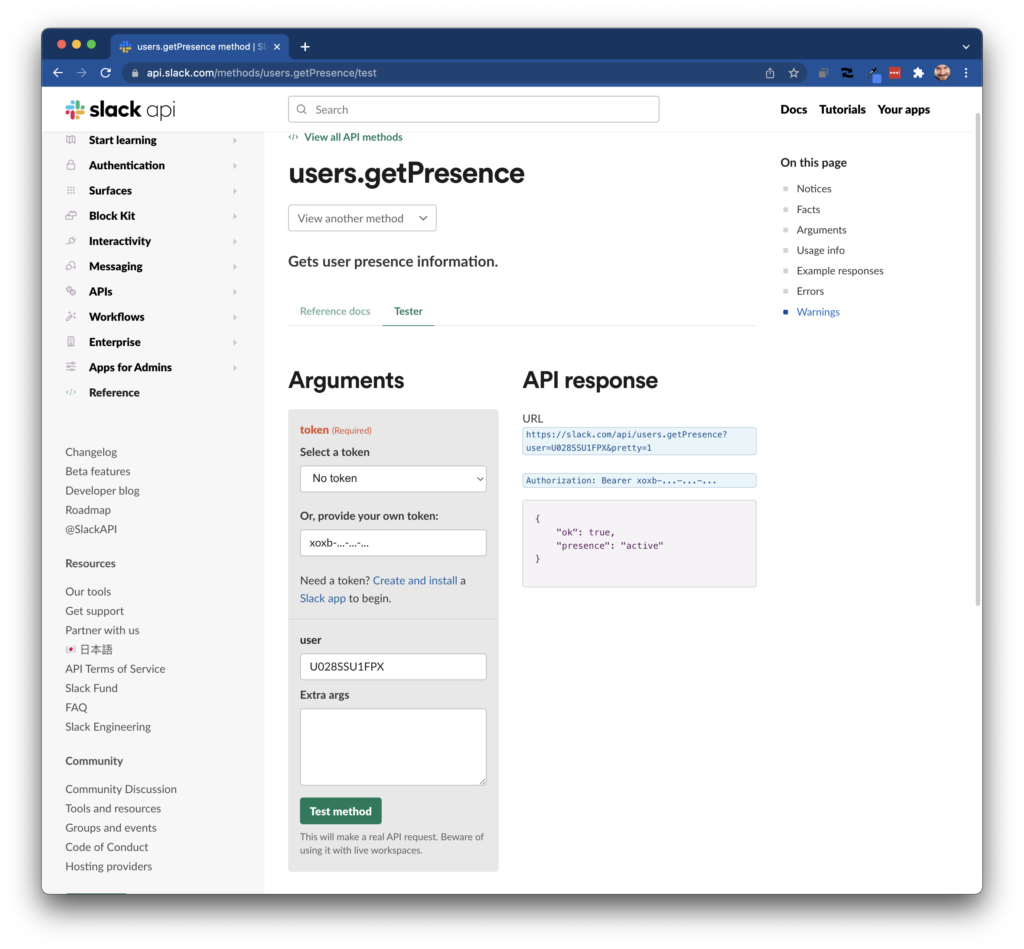
Then click on Test Method. From there, you’ll see some information under the API response including a JSON response. Depending on the user, you’ll see something similar to this:
{
"ok": true,
"presence": "active"
}
Now, let’s test another method in Insomnia.
Testing in a REST Client
In our REST API client, we’re going to use the users.list
API function which will give us a list of all of our workspace members. Further, we can use Insomnia to navigate the JSON.
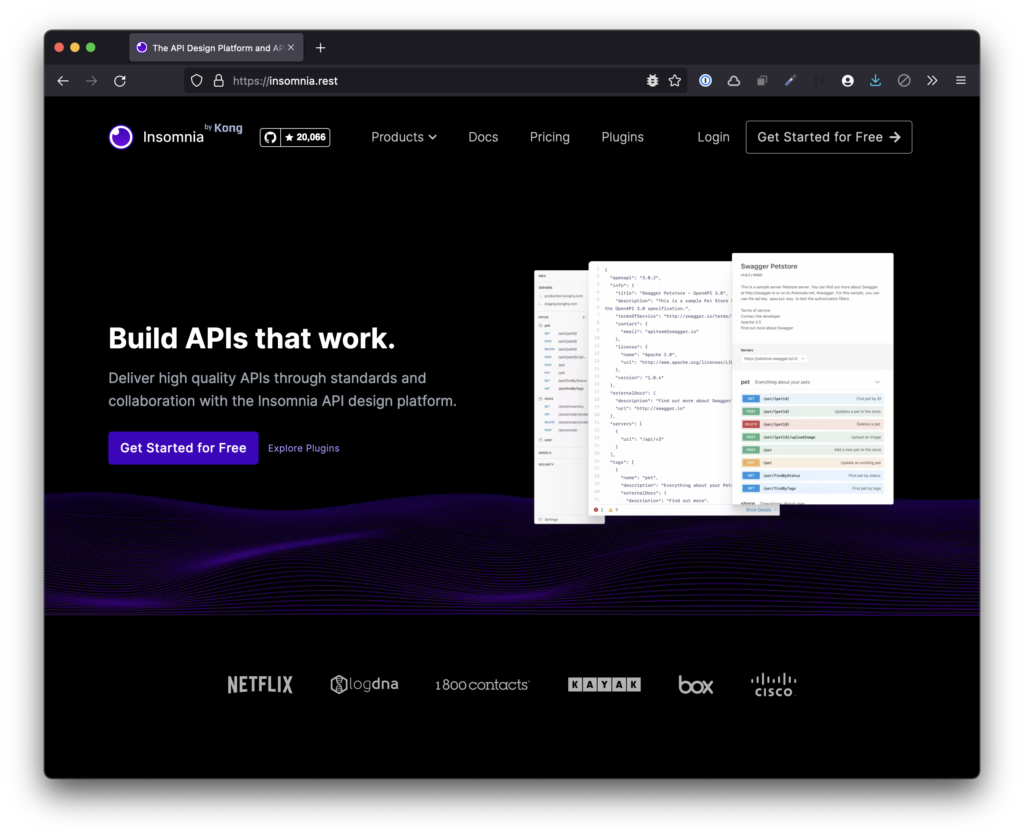
You’ll need the following:
- The API endpoint,
- What type of request we’re making,
- The OAuth Token
From there, we’ll take care of the rest in Insomnia. As an example, I’m make a request to users.list
with my token. The response is long (and yours will be based on the size of your organization).
It should look something like this:
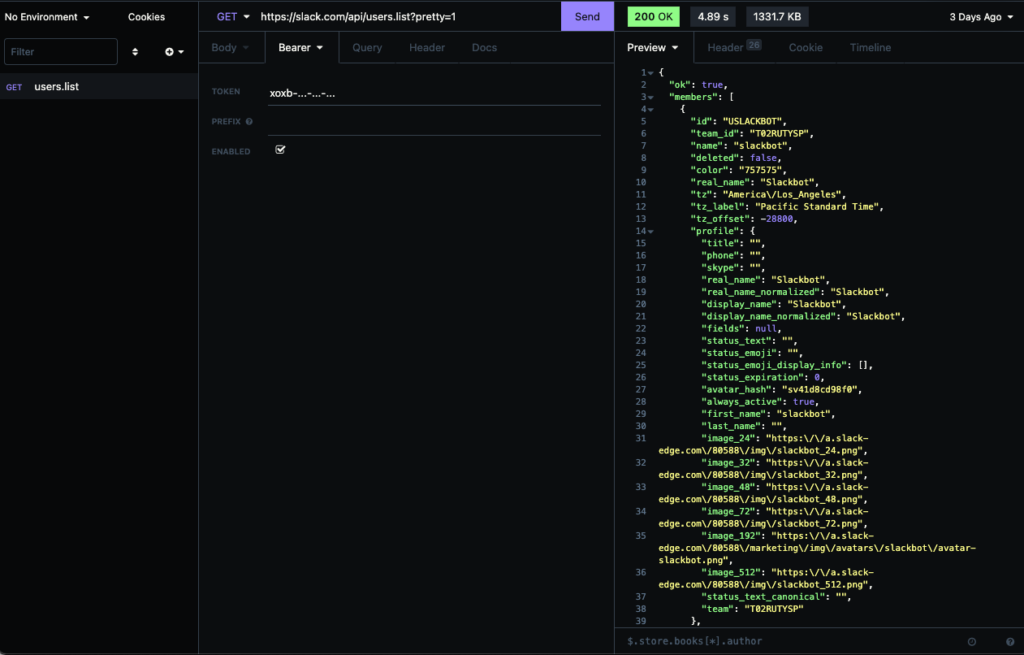
After that, we can easily navigate the JSON using the tools Insomnia provides. For example, let’s say we want to get all of the profile
nodes. We’d enter something like this:
$.members..profile
Or if we wanted to get all of the IDs for the user in the organization, then we’d enter this:
$.members..id
This will return a array of comma-delimited strings with user IDs with which you can do with it as you please.
That’s The Gist of It
From here, you know how to make the request and then can easily handle JSON within PHP or whatever language with which you’re working.