One of the things that’s always been somewhat of a point of pain in both theme and plugin development is how to handle JavaScript in WordPress.
By this, I’m not talking about third-party dependencies such as jQuery, FitVids, or whatever libraries Bootstrap, Foundation, or what’s contained within the frontend framework you opt to use when building your theme – instead, I’m talking specifically about code that we write in order to get things done within the context of our work.
When it comes to procedural programming in WordPress – think working in functions.php
– it’s expected that we’re going to be naming our functions with a unique prefix in order to prevent conflicts with other functions that may exist within plugins, third-party libraries, or even in WordPress itself.
For anyone who is just getting started in working in WordPress, this can be a hard lesson learned depending on if you’re one of the “read-the-documentation-first” type of people or not, but the thing is that the global nature of PHP mixed with the wide array of functions included in WordPress, PHP, and third-party code can lead to naming collisions that will either break the overall application or cause erratic behavior.
Most likely the former, but whatever.
But look at that: I spent the entire first part of this article talking specifically about naming PHP functions – but this is exactly the point I’m trying to make: We spend a lot of time talking about doing this in PHP, but not a lot of time talking about doing it in JavaScript.
The State Of JavaScript in WordPress
To be clear, part of what I’m saying is simply this: We need to be more careful with how we name our JavaScript functions so that we don’t accidentally end up with a naming collision just like we might in PHP.
After all, there are a quite a few similarities between JavaScript and PHP one of the biggest being the global namespace. For example, let’s say that you’re using a plugin that includes some JavaScript with a function called truncate_post_title
. The idea behind the plugin’s JavaScript code is to shorten to the post title so that it, say, only takes up one line (this is arbitrary and a weak example, but bear with me :).
Then, let’s say that you come and write your own function in JavaScript that’s intended to truncate the post title on the single post page and is going to perform some type of manipulation of the title
element’s text (again, a weird, contrived example, but hang in) and you opt to name your function truncate_post_title
.
What happens?
And that’s exactly my point. Regardless of which function fires first, only one of them is going to be “seen” by the JavaScript interpreter and it’s going to be the one doing the work.
Clearly, this can have unintended consequences.
A Potential Solution to an Anti-Pattern
One easy solution is to take all of the functions in your JavaScript source and give them a unique prefix just like you may do whenever you’re working in PHP.
Another alternative would be to wrap all of the functions within an anonymous function that you can invoke when the script is loaded, or define your functionality within a closure.
The problem that I see with the above – and this is coming from both writing code like this myself as well as working with code from others – is that if the code has too much functionality (a weird thought, I know), then it can become really difficult to trace and to debug.
That’s exactly what we should want to avoid.
So the more that I’ve been experiencing this, and the more that I’ve begun to realize (read: feel the pain) of this – again in my own code just as much as anyone else’s – the more I’ve begun to think that perhaps there needs to be a type of system or pattern that’s used when working with JavaScript in WordPress.
To keep the remainder of the article short, I’ll talk specifically about doing something like this in a plugin, but the idea can still be implemented within theme’s, as well.
Get Out of the Mud
For those who have been writing plugins for sometime and who are comfortable with jQuery, it’s not at all unlikely that your source code normally starts off with the ready
function, contains a ton of functionality wrapped within it.
Perhaps you then define some functions in the file that are called from within the anonymous function when the script begins its execution, but generally speaking, this is a format that’s popular.
The problem with this is that it can lead to a massive amount of code within the $( document ).ready
function, and/or it may result in a giant JavaScript file that contains the $( document ).ready
function as well as a number of different functions defined throughout the file that are invoked at different times.
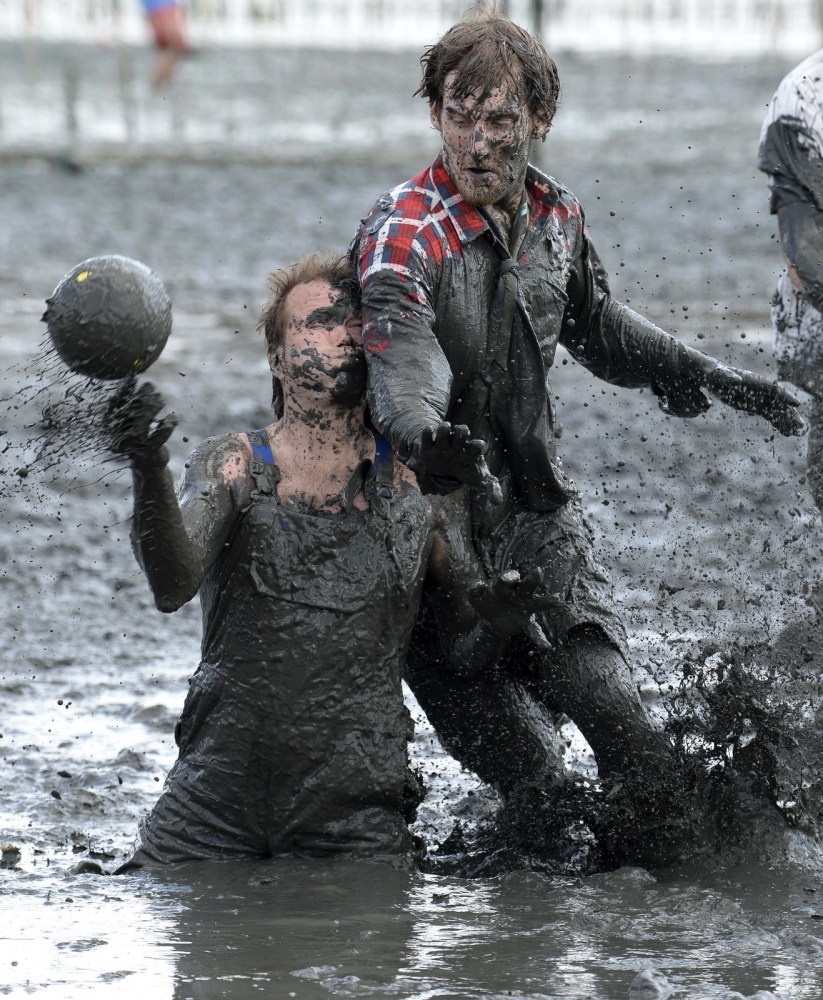
This is us. WordPress Developers. we are writing JavaScript. it is our big ball of mud.
Whatever the case, it can quickly start to feel like a big ball of mud. Ew.
A Potential Pattern
As such, one of the things that I’ve been toying with in a recent project is how to organize the JavaScript so that the code that’s initially executed is easy to read, small, and easy to trace. In order to achieve that, though, it requires additional JavaScript files that help break up the functionality into more cohesive functions.
But this still leaves one problem: How do you organize the code to prevent naming collisions? The way that I’ve been doing this – at least for now – is to use objects within their own namespace (which, in JavaScript, is basically an object within an object or an object that has another object as a property).
I’ll show some example code in a minute, but all of this has got me thinking about creating a definitive pattern that can be reused whenever we’re working on WordPress plugins (or themes). That is, there’s a bit of structure that can be reused and followed in order to prevent anti-patterns, to prevent spaghetti code, to prevent confusion when working with the code, and to prevent naming conventions.
Here’s how I’ve been thinking about it in its very simplest form:
- The Bootstrap File. Each JavaScript component – be it for the dashboard or for the public-facing side of a site – includes a bootstrap file (I normally name these `admin.js` and `public.js` that’s responsible for, say, defining the `ready` function, instantiating the rest of the variables that we need an setting things in motion.
- A Namespace. Again, in JavaScript, this is practically a wrapper object that’s used to container another object. The namespace should be unique in order to prevent collisions (that is, don’t go with the `wp` namespace. Instead, go with `Acme` or whatever the name of your company or plugin is).
- A Unique Object Name. Within the context of writing plugins, let’s say that you’re working on a widget – then perhaps the unique object name would be the name of the widget and it would be defined within the namespace.
I’ll share some example code momentarily, but the overall point (for those who are getting tired of reading) that I’m trying to make is this: I think that breaking up our JavaScript files into a bootstrap, a namespace, and uniquely named objects can go a long way in writing much more maintainable JavaScript.
The Implementation of the Pattern
So, practically, what would this look like?
Yesterday, I shared that I’ve been working on a really simple plugin. In the codebase or this plugin, I decided to put the aforementioned approach to work and ended up with something like this.
Obviously, I’ve truncated the code for ease of readability but the point remains. I’ll show the implementation of this particular pattern in reverse, because I think it makes more sense to read it that way:
First, the unique object. Note that this is contained within an outer object, one that serves as a namespace:
Secondly, the namespace:
And finally, the bootstrap file:
I assume this is easy enough to follow. I know that it leaves a lot of core functionality out, but the point of this isn’t to show how to do anything with an image widget. Instead, it’s about how to implement a potential pattern for writing cleaner, more maintainable JavaScript.
Is This Enough?
If not, I’m all for discussion as this is something that I’d love to improve if for no other reason than personally get better at what I’m doing. To that end, I’m open to whatever comments you have to offer about the above approach.
I’ve found it to be much easier to deal with than one giant JavaScript file, but I still think that there’s room to improvement this even further.
Right now, though, this is what I’ve got.
Leave a Reply
You must be logged in to post a comment.