Anytime sometime starts to get into more advanced programming – be it in WordPress or any other framework, library, foundation, or programming language – there are times in which new concepts can often be more difficult to understand than others.
I generally have found this to be true whenever a person has learned the basics of, say, object-oriented programming but hasn’t been exposed to the nuances of certain things such as design patterns.
Case in point: I’ve written about the event-driven design pattern (or the publish-subscribe or Pub/Sub as some like to refer to it) in other posts.
Yes, there are some differences to each, but the general idea is that something happens and an event is raised and anything listening for that event, or subscribed to that event, will respond.
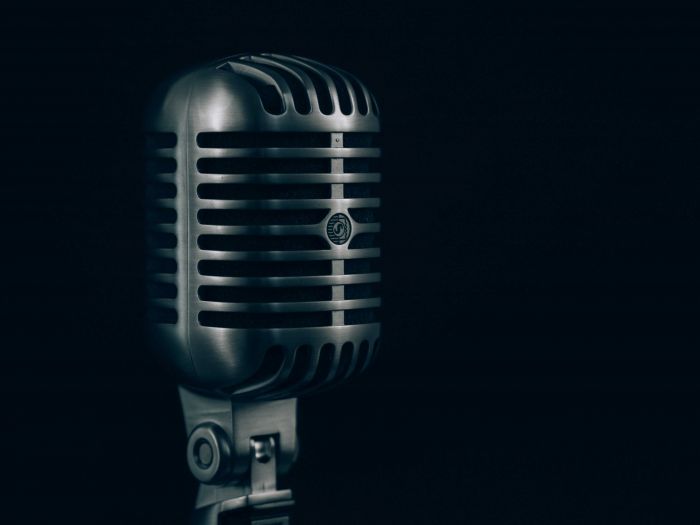
Photo by Claus Grünstäudl on Unsplash
This is the primary pattern that WordPress employs that allows us to quite literally hook into certain points of execution. We can generally conceptualize these as action hooks in WordPress.
Anyway, the application makes certain points available for us to add our own functionality. Once that functionality is registered, WordPress will leave its codebase, so to speak, hop into ours, then return back to ours.
It’s easy enough to understand, but what if you want to expose areas in your code that allow others to hook into your code?
Action Hooks in WordPress
Before looking at how WordPress implements this patterns, it’s important to understand the basics of this pattern. Though this is by no means comprehensive, it’s meant to help give a foundational understanding of the pattern so that it’s possible to read and write WordPress-centric code.
So what is a good way to think of the Pub/Sub Pattern? Wikipedia defines it as:
In software architecture, publish–subscribe is a messaging pattern where senders of messages, called publishers, do not program the messages to be sent directly to specific receivers, called subscribers, but instead categorize published messages into classes without knowledge of which subscribers, if any, there may be. Similarly, subscribers express interest in one or more classes and only receive messages that are of interest, without knowledge of which publishers, if any, there are.
Understanding the Pattern
This might be a lot to take in at first. I don’t know, but let’s break it down:
- There is a service, in our case being WordPress, that’s responsible for publishing messages out to whoever is subscribing (it doesn’t necessarily know who is listening).
- When a subscriber is listening, it will then take action whenever it hears that action.
- Once the subscriber’s code is done executing, the program will then return back to the original point of execution (which is where the publisher sent the message).
There are nuances to this such as asynchronous functionality and things like that, but that’s more advanced than I’d prefer to get in this particular post. After all, the purpose of this is to lay a foundation for understanding and implementing functionality.
Asynchronous functionality can get into threading or Ajax an through those are important topics, this is not that post.
What’s This Look like in WordPress?
Perhaps the easiest way to describe this particular pattern in WordPress is through the use of the function calls:
- do_action
- add_action
Sometimes, the nomenclature can be confusing but simply put, do_action publishes and an event and add_action subscribers to an event. Or perhaps a better way of thinking of it is:
do_action tells WordPress to perform whatever actions have been added.
Sometimes having simple phrases to remember how things work is helpful. I don’t know if the above is the catchiest phrase or most memorable, but it’s something, right?
Furthermore, note that do_action and add_action are things that are core to WordPress and are also available to our development. Before going any further, let’s take a look at what each means:
For do_action:
This function invokes all functions attached to action hook
$tag
. It is possible to create new action hooks by simply calling this function, specifying the name of the new hook using the$tag
parameter.
Or even more simply put:
Execute functions hooked on a specific action hook.
When referring to hooks, this can either be hooks defined by WordPress or custom hooks that you specify in your theme or your plugin.
As for add_action:
Actions are the hooks that the WordPress core launches at specific points during execution, or when specific events occur. Plugins can specify that one or more of its PHP functions are executed at these points, using the Action API.
And, similarly, more simply put:
Hooks a function on to a specific action.
Setting this practically is a bit different because we’re generally using add_action to add our own code to WordPress.
A Practical Example
For example, perhaps you’ve written something like this:
In this case, somewhere in the WordPress codebase there’s a do_action call for the wp_insert_post_data hook and it accepts a function and will pass it at least a single parameter.
Adding Your Own Hooks
But what if you want to give other developers to ability to hook into your plugin or theme? In that case, you should concern yourself with using do_action and the page linked earlier in this document provides everything you need to set this up.
It’s actually far simpler, in my opinion, than working with add_action because add_action provides that we not only hook into an existing publisher, but that we add our own custom logic.
do_action on the other hand requires that we provide a name of the function to be executed and then the list of arguments to be passed to the function that will be executed.
That’s It?
In about as simple terms as I can make it, yes. There are some nuances around priority, number of arguments, and working with namespaces and object-oriented programming. But, again, that’s outside the scope of this particular post. Perhaps I’ll at it more in-depth in another post.
For now, though, if you’re not familiar with the basics of:
- the Pub/Sub Pattern,
- do_action,
- and add_action
You’re now comfortable enough to read the code you’re working with, understanding how the code is functioning, and even implement your own solutions when needed.